火车购票系统 登录注册功能实现.用户登录管理员登录 用户购票管理员操作: 1.界面显示: 进入界面应当有选项1添加车票,选项2删除车票,选项3更新车票,选项4查询车票,选项 5退出登录(基础选项)车票信息应当以1,2,3列表形式直接展示。添加车票功能实现,删除车票功能实现,更新车票功能实现,查询车票功能实现在进行任意操作时,按任意字符不会导致系统崩溃,代码实现,必须用类实现(含属性,方法)用c++实现
时间: 2023-11-18 18:02:11 浏览: 181
好的,以下是使用 C++ 实现火车购票系统的登录和注册功能,以及管理员操作的示例代码:
```c++
#include <iostream>
#include <iomanip>
#include <vector>
#include <cstring>
using namespace std;
// 用户类
class User {
public:
string username;
string password;
bool is_admin;
User(string username, string password) {
this->username = username;
this->password = password;
this->is_admin = false;
}
};
// 管理员类,继承自用户类
class Admin : public User {
public:
Admin(string username, string password) : User(username, password) {
this->is_admin = true;
}
};
// 车票类
class Ticket {
public:
string id;
string name;
double price;
int count;
Ticket(string id, string name, double price, int count) {
this->id = id;
this->name = name;
this->price = price;
this->count = count;
}
};
// 火车购票系统类
class TrainTicketSystem {
private:
vector<User*> users; // 所有用户列表
vector<Admin*> admins; // 所有管理员列表
vector<Ticket*> tickets; // 所有车票列表
User* current_user; // 当前登录用户
public:
TrainTicketSystem() {
this->current_user = nullptr;
}
// 注册功能实现
void register_user(string username, string password) {
User* user = new User(username, password);
for (auto u : this->users) {
if (u->username == username) {
cout << "注册失败,该用户已存在!" << endl;
return;
}
}
this->users.push_back(user);
cout << "注册成功!" << endl;
}
// 登录功能实现
void login(string username, string password) {
for (auto user : this->users) {
if (user->username == username && user->password == password) {
this->current_user = user;
cout << "登录成功!" << endl;
return;
}
}
cout << "登录失败,用户名或密码错误!" << endl;
}
// 管理员登录功能实现
void admin_login(string username, string password) {
for (auto admin : this->admins) {
if (admin->username == username && admin->password == password) {
this->current_user = admin;
cout << "管理员登录成功!" << endl;
return;
}
}
cout << "管理员登录失败,用户名或密码错误!" << endl;
}
// 添加车票功能实现
void add_ticket(string id, string name, double price, int count) {
if (!this->current_user || !this->current_user->is_admin) {
cout << "添加车票失败,需要管理员权限!" << endl;
return;
}
Ticket* ticket = new Ticket(id, name, price, count);
this->tickets.push_back(ticket);
cout << "添加车票成功!" << endl;
}
// 删除车票功能实现
void remove_ticket(string id) {
if (!this->current_user || !this->current_user->is_admin) {
cout << "删除车票失败,需要管理员权限!" << endl;
return;
}
for (auto iter = this->tickets.begin(); iter != this->tickets.end(); iter++) {
if ((*iter)->id == id) {
this->tickets.erase(iter);
cout << "删除车票成功!" << endl;
return;
}
}
cout << "删除车票失败,不存在该车票!" << endl;
}
// 更新车票功能实现
void update_ticket(string id, string name="", double price=0, int count=0) {
if (!this->current_user || !this->current_user->is_admin) {
cout << "更新车票失败,需要管理员权限!" << endl;
return;
}
for (auto ticket : this->tickets) {
if (ticket->id == id) {
if (name != "") {
ticket->name = name;
}
if (price != 0) {
ticket->price = price;
}
if (count != 0) {
ticket->count = count;
}
cout << "更新车票成功!" << endl;
return;
}
}
cout << "更新车票失败,不存在该车票!" << endl;
}
// 查询车票功能实现
void query_ticket() {
if (this->tickets.empty()) {
cout << "当前没有车票记录!" << endl;
return;
}
cout << "车票信息:" << endl;
cout << "编号\t\t名称\t\t价格\t\t数量" << endl;
for (auto ticket : this->tickets) {
cout << ticket->id << "\t\t" << ticket->name << "\t\t" << fixed << setprecision(2) << ticket->price << "\t\t" << ticket->count << endl;
}
}
// 退出登录功能实现
void logout() {
this->current_user = nullptr;
cout << "退出登录成功!" << endl;
}
};
int main() {
TrainTicketSystem system;
// 添加管理员账号
Admin* admin = new Admin("admin", "admin");
system.admins.push_back(admin);
while (true) {
cout << "欢迎使用火车购票系统,请选择操作:" << endl;
cout << "1. 注册" << endl;
cout << "2. 登录" << endl;
cout << "3. 管理员登录" << endl;
cout << "4. 添加车票" << endl;
cout << "5. 删除车票" << endl;
cout << "6. 更新车票" << endl;
cout << "7. 查询车票" << endl;
cout << "8. 退出登录" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1: {
cout << "请输入用户名和密码:" << endl;
string username, password;
cin >> username >> password;
system.register_user(username, password);
break;
}
case 2: {
cout << "请输入用户名和密码:" << endl;
string username, password;
cin >> username >> password;
system.login(username, password);
break;
}
case 3: {
cout << "请输入管理员用户名和密码:" << endl;
string username, password;
cin >> username >> password;
system.admin_login(username, password);
break;
}
case 4: {
if (!system.current_user) {
cout << "请先登录!" << endl;
break;
}
cout << "请输入车票编号,名称,价格和数量:" << endl;
string id, name;
double price;
int count;
cin >> id >> name >> price >> count;
system.add_ticket(id, name, price, count);
break;
}
case 5: {
if (!system.current_user) {
cout << "请先登录!" << endl;
break;
}
cout << "请输入要删除的车票编号:" << endl;
string id;
cin >> id;
system.remove_ticket(id);
break;
}
case 6: {
if (!system.current_user) {
cout << "请先登录!" << endl;
break;
}
cout << "请输入要更新的车票编号,可选更新项为名称,价格和数量(不需要更新的项请直接回车):" << endl;
string id, name;
double price;
int count;
cin >> id >> name >> price >> count;
system.update_ticket(id, name, price, count);
break;
}
case 7: {
system.query_ticket();
break;
}
case 8: {
system.logout();
break;
}
default: {
cout << "无效的选择,请重新输入!" << endl;
break;
}
}
}
return 0;
}
```
这段代码中,我们同样定义了用户类、管理员类、车票类和火车购票系统类。在火车购票系统类中,我们使用 STL 容器 `vector` 存储所有用户、管理员和车票记录,以及记录当前登录用户的信息。登录和注册功能的实现比较简单,主要是遍历用户列表查找匹配的用户信息。管理员操作需要检查当前登录用户是否为管理员,以此判断是否有权限进行操作。具体的功能实现比较清晰,代码中有详细的注释说明。
阅读全文
相关推荐
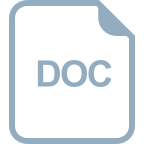
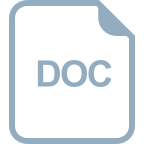
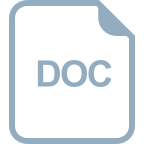


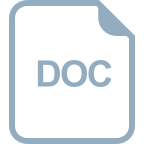
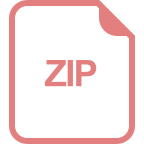
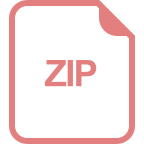
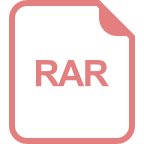
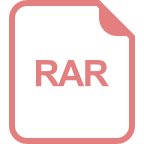
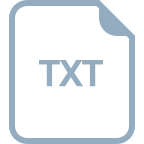
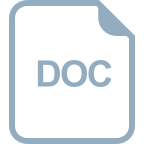
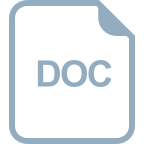
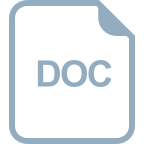
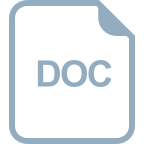
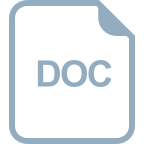
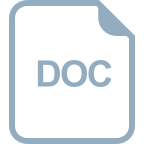
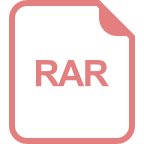