jquery post header
时间: 2023-07-27 10:07:18 浏览: 143
如果你想在 jQuery POST 请求中添加自定义的 HTTP 头部,可以使用 jQuery AJAX 的 `headers` 选项。
示例代码如下:
```
$.ajax({
url: 'your_api_url',
method: 'POST',
headers: {
'Authorization': 'Bearer Your_Access_Token',
'Content-Type': 'application/json'
},
data: {
// your data here
},
success: function(response) {
// handle success response
},
error: function(jqXHR, textStatus, errorThrown) {
// handle error response
}
});
```
在上面的代码中,`headers` 对象包含了两个自定义的 HTTP 头部,即 `Authorization` 和 `Content-Type`。你可以根据你的实际需要添加自定义的 HTTP 头部。
相关问题
Access to XMLHttpRequest at 'http://localhost/imovie/view/details.php' from origin 'null' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. jquery.js:2 POST http://localhost/imovie/view/details.php net::ERR_FAILED 404 (Not Found) send @ jquery.js:2 ajax @ jquery.js:2 k.<computed> @ jquery.js:2 (匿名) @ home.html:300 dispatch @ jquery.js:2 v.handle @ jquery.js:2
这个错误是因为在你的 JavaScript 代码中,你正在尝试从一个不同的域名或端口号发送 AJAX 请求。由于安全原因,浏览器会阻止这样的请求。你需要在服务器端设置跨域资源共享(CORS)响应头,以允许从其他域名或端口号访问你的服务器资源。在 PHP 中可以通过设置响应头来解决这个问题:
```
header("Access-Control-Allow-Origin: *");
```
在这里,我们将 `*` 作为允许访问的域名,这意味着允许任何域名访问你的资源。如果你希望只允许特定的域名访问你的资源,可以将 `*` 替换为相应的域名。
jquery mobile 下拉框post异步获取数据的例子
以下是一个基本的示例,演示如何使用jQuery Mobile下拉框和AJAX从服务器异步检索数据。
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>jQuery Mobile 下拉框异步获取数据示例</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.css">
<script src="https://code.jquery.com/jquery-1.11.3.min.js"></script>
<script src="https://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.js"></script>
</head>
<body>
<div data-role="page">
<div data-role="header">
<h1>下拉框异步获取数据示例</h1>
</div>
<div data-role="main" class="ui-content">
<label for="select-choice-1" class="select">选择一个城市:</label>
<select name="select-choice-1" id="select-choice-1">
<option value="">请选择...</option>
<option value="1">北京</option>
<option value="2">上海</option>
<option value="3">广州</option>
<option value="4">深圳</option>
</select>
<div id="result"></div>
</div>
<div data-role="footer">
<h4>版权所有 © 2021</h4>
</div>
</div>
<script>
$(document).on("pagecreate", function() {
$("#select-choice-1").on("change", function() {
var cityId = $("#select-choice-1").val();
$.ajax({
url: "getdata.php",
type: "POST",
data: { city_id: cityId },
dataType: "html",
success: function(data) {
$("#result").html(data);
}
});
});
});
</script>
</body>
</html>
```
JavaScript代码:
```javascript
$(document).on("pagecreate", function() {
$("#select-choice-1").on("change", function() {
var cityId = $("#select-choice-1").val();
$.ajax({
url: "getdata.php", // 服务器端处理程序的URL地址
type: "POST", // 请求方式
data: { city_id: cityId }, // 发送到服务器端的数据
dataType: "html", // 服务器端返回的数据类型
success: function(data) { // 成功返回数据后的回调函数
$("#result").html(data); // 将返回的数据显示在页面上
}
});
});
});
```
PHP代码(getdata.php):
```php
<?php
if(isset($_POST['city_id'])) {
$cityId = $_POST['city_id'];
if($cityId == '1') {
echo '<p>您选择了北京。</p>';
} else if($cityId == '2') {
echo '<p>您选择了上海。</p>';
} else if($cityId == '3') {
echo '<p>您选择了广州。</p>';
} else if($cityId == '4') {
echo '<p>您选择了深圳。</p>';
}
}
?>
```
在这个示例中,我们创建了一个简单的HTML页面,其中包含一个下拉框和一个用于显示结果的div元素。当用户选择下拉框中的选项时,我们使用jQuery的AJAX函数从服务器异步检索数据,并将结果显示在页面上。
请注意,我们使用了jQuery的on()函数来监听页面创建事件,以便在页面加载时绑定事件处理程序。在事件处理程序中,我们使用jQuery的ajax()函数向服务器发送POST请求,并传递所选城市的ID作为数据。
服务器通过检查POST请求中的城市ID参数来确定要返回的数据,然后将数据返回到客户端。在客户端,我们在ajax()函数的成功回调函数中处理返回的数据,并将其显示在页面上。
以上就是一个基本的jQuery Mobile下拉框post异步获取数据的例子。
阅读全文
相关推荐
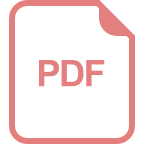
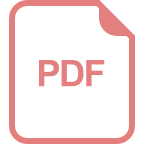
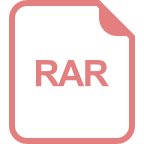
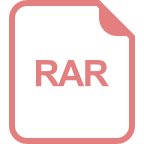
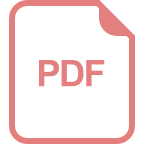
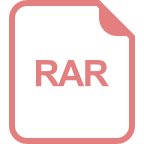
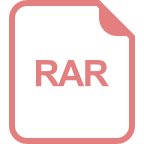
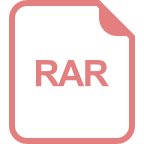
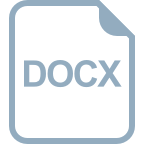
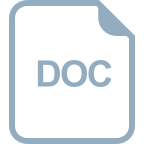
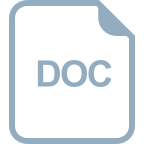
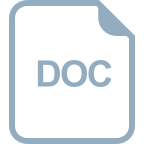
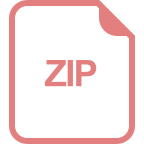