def generate_paths(t, value): """Yields all possible paths from the root of t to a node with the label value as a list. >>> t1 = Tree(1, [Tree(2, [Tree(3), Tree(4, [Tree(6)]), Tree(5)]), Tree(5)]) >>> print(t1) 1 2 3 4 6 5 5 >>> next(generate_paths(t1, 6)) [1, 2, 4, 6] >>> path_to_5 = generate_paths(t1, 5) >>> sorted(list(path_to_5)) [[1, 2, 5], [1, 5]] >>> t2 = Tree(0, [Tree(2, [t1])]) >>> print(t2) 0 2 1 2 3 4 6 5 5 >>> path_to_2 = generate_paths(t2, 2) >>> sorted(list(path_to_2)) [[0, 2], [0, 2, 1, 2]] """ "*** YOUR CODE HERE ***" for _______________ in _________________: for _______________ in _________________: "*** YOUR CODE HERE ***"
时间: 2024-03-03 10:50:17 浏览: 26
这是一道编程题,需要填写代码实现函数generate_paths(t, value)的功能,该函数的作用是返回从树t的根节点到值为value的所有可能路径。
以下是代码填空部分:
```
def generate_paths(t, value):
path = [t.label]
if t.label == value:
yield path
for branch in t.branches:
for subpath in generate_paths(branch, value):
yield path + subpath
```
解释:
首先定义了一个辅助函数path,用来记录当前路径。然后判断当前节点的值是否等于value,如果是则yield当前路径。接着递归遍历每个子树,对于每个子树,都执行一个循环,循环中递归调用generate_paths函数得到子树中值为value的路径subpath,并将当前路径path与subpath合并,得到完整的路径,然后yield出去。
相关问题
Define a generator function generate_paths which takes in a Tree t, a value value, and returns a generator object which yields each path from the root of t to a node that has label value. t is implemented with a class, not as the function-based ADT. Each path should be represented as a list of the labels along that path in the tree. You may yield the paths in any order. We have provided a (partial) skeleton for you. You do not need to use this skeleton, but if your implementation diverges significantly from it, you might want to think about how you can get it to fit the skeleton.其中class Tree: def init(self, label, branches=[]): for b in branches: assert isinstance(b, Tree) self.label = label self.branches = list(branches) def is_leaf(self): return not self.branches def map(self, fn): self.label = fn(self.label) for b in self.branches: b.map(fn) def contains(self, e): if self.label == e: return True for b in self.branches: if e in b: return True return False def repr(self): if self.branches: branch_str = ', ' + repr(self.branches) else: branch_str = '' return 'Tree({0}{1})'.format(self.label, branch_str) def str(self): def print_tree(t, indent=0): tree_str = ' ' * indent + str(t.label) + "\n" for b in t.branches: tree_str += print_tree(b, indent + 1) return tree_str return print_tree(self).rstrip()
以下是实现 generate_paths 函数的代码:
```
def generate_paths(t, value):
if t.label == value:
yield [t.label]
for b in t.branches:
for path in generate_paths(b, value):
yield [t.label] + path
```
这个函数首先检查当前节点的标签是否为目标值 value,如果是,则生成一个只包含当前节点标签的路径。然后遍历当前节点的所有分支,递归调用 generate_paths 函数,并将每个返回的路径前面加上当前节点的标签,生成从当前节点到目标节点的所有路径。由于该函数是使用生成器实现的,因此可以逐步生成所有路径,而不是一次性生成所有路径,从而优化内存使用。注意,路径的顺序是不确定的,因此可以按任意顺序返回路径。
Generate a column vector y with the same number of elements as x that has evenly spaced values ranging from the minimum value in x to the maximum value in x.
您可以使用MATLAB中的linspace函数来生成一个列向量y,该向量具有与x相同的元素数量,其值从x中的最小值到最大值之间均匀分布。具体代码如下:
y = linspace(min(x), max(x), numel(x))'
相关推荐
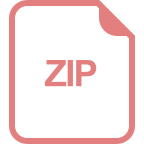
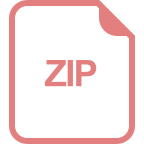













