利用C#设计一个应用软件连接数据库,设计一个购物系统
时间: 2024-01-15 16:05:24 浏览: 91
好的,下面为您提供一个基本的购物系统设计:
首先,需要连接数据库,可以使用C#自带的ADO.NET技术,具体步骤如下:
1. 在Visual Studio中,新建一个Windows Form应用程序项目。
2. 在“解决方案资源管理器”中,右键单击“引用”,选择“添加引用”,在“添加引用”对话框中选择“System.Data.dll”并添加。
3. 在窗口中添加一个DataGridView控件,用于显示商品列表。
4. 添加一个“连接”按钮和一个“查询”按钮,并分别设置它们的Click事件。
5. 在连接按钮的Click事件中,编写连接数据库的代码,如下所示:
```csharp
using System.Data.SqlClient;
...
private void btnConnect_Click(object sender, EventArgs e)
{
try
{
SqlConnection conn = new SqlConnection("Data Source=.;Initial Catalog=ShopDB;Integrated Security=True");
conn.Open();
MessageBox.Show("数据库连接成功!");
conn.Close();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
```
这里使用了SQL Server作为数据库,Data Source表示服务器名,Initial Catalog表示数据库名,Integrated Security=True表示使用Windows身份验证方式登录数据库。
6. 在查询按钮的Click事件中,编写查询商品列表的代码,如下所示:
```csharp
private void btnQuery_Click(object sender, EventArgs e)
{
try
{
SqlConnection conn = new SqlConnection("Data Source=.;Initial Catalog=ShopDB;Integrated Security=True");
conn.Open();
SqlDataAdapter da = new SqlDataAdapter("select * from Product", conn);
DataTable dt = new DataTable();
da.Fill(dt);
dataGridView1.DataSource = dt;
conn.Close();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
```
这里使用了SqlDataAdapter类来填充DataTable,然后将DataTable绑定到DataGridView控件上。
接下来,设计购物系统的界面和功能:
1. 在窗口中添加一个ListBox控件,用于显示购物车中的商品列表。
2. 在DataGridView控件的CellDoubleClick事件中,编写将商品加入购物车的代码,如下所示:
```csharp
private void dataGridView1_CellDoubleClick(object sender, DataGridViewCellEventArgs e)
{
try
{
string productName = dataGridView1.Rows[e.RowIndex].Cells["ProductName"].Value.ToString();
decimal price = decimal.Parse(dataGridView1.Rows[e.RowIndex].Cells["Price"].Value.ToString());
listBox1.Items.Add(productName + " - " + price.ToString("c"));
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
```
这里获取选中行的商品名称和价格,然后将它们加入到购物车中。
3. 在ListBox控件的DoubleClick事件中,编写将商品从购物车中移除的代码,如下所示:
```csharp
private void listBox1_DoubleClick(object sender, EventArgs e)
{
try
{
if (listBox1.SelectedIndex != -1)
{
listBox1.Items.RemoveAt(listBox1.SelectedIndex);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
```
4. 添加一个“结算”按钮,并在它的Click事件中编写计算总金额的代码,如下所示:
```csharp
private void btnCheckout_Click(object sender, EventArgs e)
{
try
{
decimal total = 0;
foreach (string item in listBox1.Items)
{
string[] parts = item.Split('-');
decimal price = decimal.Parse(parts[1].Trim());
total += price;
}
MessageBox.Show("总金额为:" + total.ToString("c"));
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
```
这里遍历购物车中的商品,计算总金额并弹出提示框显示。
至此,一个简单的购物系统就设计完成了。当然,实际的购物系统肯定还需要更多的功能和细节处理,比如购物车中数量的增减、商品的分类和搜索、结算时的优惠和支付方式等等。
阅读全文
相关推荐
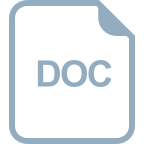
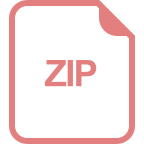
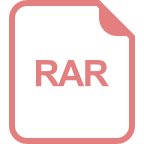
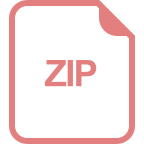
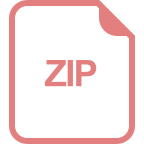
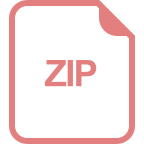
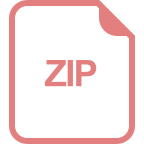
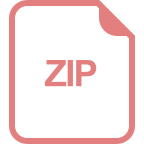
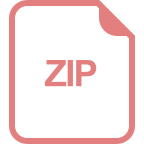
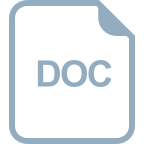
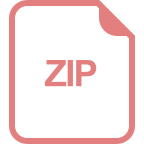
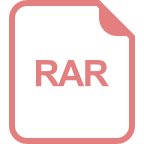
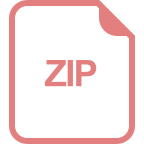
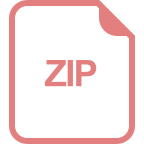
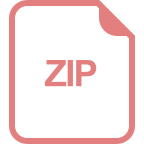
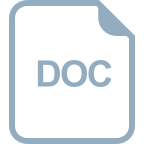
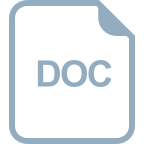