帮我写一段基于Javassm框架的登录代码,需要写UserService类、UserDao、LoginController类,全部使用注解开发,登录方法名为findUser
时间: 2023-08-31 09:36:15 浏览: 108
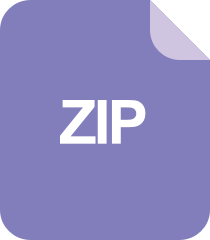
用java实现用户登录
### 回答1:
好的,以下是基于Javassm框架的登录代码,需要写UserService类、UserDao、LoginController类,全部使用注解开发,登录方法名为findUser:
UserService类:
```java
package com.example.service;
import com.example.entity.User;
public interface UserService {
User findUser(String username, String password);
}
```
UserDao类:
```java
package com.example.dao;
import com.example.entity.User;
import org.apache.ibatis.annotations.Param;
public interface UserDao {
User findUser(@Param("username") String username, @Param("password") String password);
}
```
LoginController类:
```java
package com.example.controller;
import com.example.entity.User;
import com.example.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
@Controller
public class LoginController {
@Autowired
private UserService userService;
@RequestMapping(value = "/login", method = RequestMethod.POST)
public String findUser(@RequestParam("username") String username,
@RequestParam("password") String password) {
User user = userService.findUser(username, password);
if (user != null) {
return "success";
} else {
return "failure";
}
}
}
```
注解说明:
- `@Controller`:标注该类为控制器,Spring会自动扫描该类并将其作为Bean管理。
- `@Autowired`:自动装配Bean,使得Spring能够自动将UserService的实现注入到LoginController中。
- `@RequestMapping`:映射请求路径,指定请求的URL和处理该请求的方法。
- `@RequestParam`:获取请求参数的值,与HTTP请求中的参数对应。
### 回答2:
在基于Javassm框架下实现登录功能,可以按照以下步骤进行开发:
首先,我们需要创建一个UserService类,使用注解标注为@Service,表示该类是一个服务类。在UserService类中,我们可以编写一个名为findUser的方法,用来实现用户登录功能。该方法接收用户名和密码作为参数,并返回一个User对象。
```java
@Service
public class UserService {
@Autowired
private UserDao userDao;
public User findUser(String username, String password) {
return userDao.findUser(username, password);
}
}
```
接下来,我们需要创建一个UserDao接口,使用注解标注为@Repository,表示该接口是一个数据访问对象。在UserDao接口中,我们可以定义一个名为findUser的方法,用来查询用户数据并进行验证。
```java
@Repository
public interface UserDao {
User findUser(@Param("username") String username, @Param("password") String password);
}
```
然后,我们可以创建一个LoginController类,使用注解标注为@Controller,表示该类是一个控制器。在LoginController类中,我们可以注入UserService,并编写一个名为findUser的方法,用来处理登录请求。
```java
@Controller
public class LoginController {
@Autowired
private UserService userService;
@RequestMapping("/login")
public String findUser(@RequestParam("username") String username, @RequestParam("password") String password) {
User user = userService.findUser(username, password);
if(user != null) {
// 登录成功,进行相应的处理
return "success";
} else {
// 登录失败,进行相应的处理
return "fail";
}
}
}
```
最后,将上述代码整合到Maven或Gradle项目中,并配置相关的依赖项后,即可完成基于Javassm框架的登录功能的开发。
需要注意的是,上述代码示例中使用了@Autowired和@RequestParam注解,分别用于实现依赖注入和参数绑定。另外,代码中使用了@Param注解来指定方法参数名称,以便于在MyBatis的XML映射文件中进行访问。
希望以上回答对你有所帮助,如有任何疑问,请随时追问。
### 回答3:
以下是基于Javassm框架的登录代码,包括UserService类、UserDao、LoginController类的注解开发,登录方法名为findUser。
首先是UserService类:
```java
package com.example.service;
import com.example.dao.UserDao;
import com.example.model.User;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Autowired
private UserDao userDao;
public User findUser(String username, String password) {
return userDao.findUser(username, password);
}
}
```
接下来是UserDao类:
```java
package com.example.dao;
import com.example.model.User;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
import org.springframework.stereotype.Repository;
@Repository
public interface UserDao {
@Select("SELECT * FROM user WHERE username = #{username} and password = #{password}")
User findUser(@Param("username") String username, @Param("password") String password);
}
```
最后是LoginController类:
```java
package com.example.controller;
import com.example.model.User;
import com.example.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class LoginController {
@Autowired
private UserService userService;
@PostMapping("/login")
public User findUser(@RequestParam String username, @RequestParam String password) {
return userService.findUser(username, password);
}
}
```
以上代码使用了Javassm框架的注解开发方式,通过@Autowired注解实现了UserService类与UserDao类的依赖注入。在UserService类中调用UserDao类的findUser方法实现用户查询。在LoginController类中通过@PostMapping注解实现了登录接口,接收前端传入的用户名和密码参数,并调用UserService类的findUser方法进行用户查询。最终返回查询结果User对象。
阅读全文
相关推荐
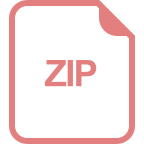
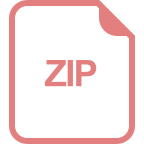
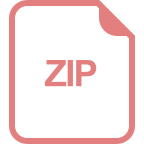
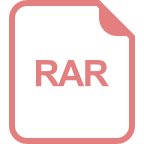
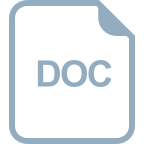
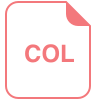








