人口增长logistic模代码
时间: 2023-09-01 12:08:40 浏览: 315
下面是一个简单的 Python 代码实现人口增长的 logistic 模型:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义模型函数
def logistic(t, K, r, P0):
P = K / (1 + ((K-P0)/P0) * np.exp(-r*t))
return P
# 定义参数
K = 10 # 最大承载量
r = 0.1 # 增长率
P0 = 1 # 初始人口数量
t = np.linspace(0, 50, 1000) # 时间范围
# 计算模型预测值
P = logistic(t, K, r, P0)
# 绘制人口增长曲线
plt.plot(t, P)
plt.xlabel('时间')
plt.ylabel('人口数量')
plt.title('人口增长的 logistic 模型')
plt.show()
```
在上面的代码中,我们通过定义模型函数 `logistic` 来实现人口增长的 logistic 模型。这个模型函数接受四个参数:最大承载量 `K`、增长率 `r`、初始人口数量 `P0` 和时间 `t`。在主程序中,我们设置了这些参数的值,并使用 `numpy` 库生成了一个时间范围。然后,我们通过调用模型函数 `logistic` 来计算出人口数量 `P` 的预测值,并使用 `matplotlib` 库绘制了人口增长曲线。
相关问题
建立人口增长logistic模型,,画出图像,给出python代码
人口增长的 Logistic 模型可以表示如下:
$$\frac{dN}{dt}=rN\left(1-\frac{N}{K}\right)$$
其中,$N$ 表示人口数量,$t$ 表示时间,$r$ 是人口增长率,$K$ 是环境容纳量。
为了画出该模型的图像,我们可以使用 Python 中的 SciPy 库中的 ode 模块进行数值求解,并使用 Matplotlib 库绘制图像。下面是 Python 代码实现:
```python
import numpy as np
from scipy.integrate import odeint
import matplotlib.pyplot as plt
# 定义人口增长模型
def logistic(N, t, r, K):
dNdt = r * N * (1 - N/K)
return dNdt
# 设置初始值
N0 = 10
t = np.linspace(0, 50, 1000)
# 定义参数
r = 0.1
K = 100
# 求解微分方程
N = odeint(logistic, N0, t, args=(r, K))
# 绘制图像
plt.plot(t, N, label='Population')
plt.xlabel('Time')
plt.ylabel('Population')
plt.title('Logistic Model')
plt.legend()
plt.show()
```
运行该代码,即可得到人口增长 Logistic 模型的图像。
人口logistic模型代码
人口Logistic模型是一种常用的数学模型,它描述了种群随时间的增长趋势,在资源有限的情况下会受到制约。这种模型假设种群增长率为该种群数量与环境容纳量的比例乘以剩余的资源空间。以下是Python中使用`scipy.optimize`库求解人口Logistic模型的一个简单示例:
```python
import numpy as np
from scipy.optimize import fsolve
# Logistic growth function
def logistic_growth(population, carrying_capacity, initial_population):
return population * (carrying_capacity - population) / carrying_capacity + initial_population - population
# 定义初始条件、资源容纳量和迭代函数参数
initial_population = 50
carrying_capacity = 1000
t_max = 100 # 观察时间范围
time_steps = 1000 # 时间间隔
# 使用fsolve求解每个时间步的人口数量
times = np.linspace(0, t_max, time_steps)
solutions = [fsolve(logistic_growth, initial_population, args=(carrying_capacity, t)) for t in times]
# 显示结果
for i, t in enumerate(times):
print(f"时间 {t:.2f}: 种群数量 {solutions[i][0]:.2f}")
阅读全文
相关推荐
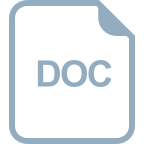



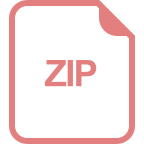
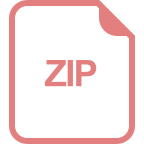
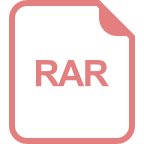
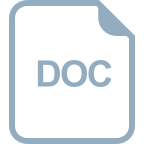
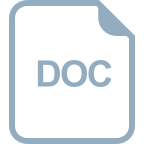
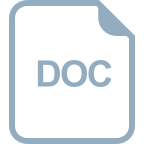



