用python实现,定时开关多荐任务
时间: 2023-02-11 07:24:55 浏览: 112
可以使用 Python 的 schedule 模块来实现定时开关多个任务。下面是一个简单的示例代码:
```python
import schedule
import time
def job1():
print("Job 1 is running.")
def job2():
print("Job 2 is running.")
def job3():
print("Job 3 is running.")
schedule.every(5).seconds.do(job1)
schedule.every(10).seconds.do(job2)
schedule.every(15).seconds.do(job3)
while True:
schedule.run_pending()
time.sleep(1)
```
这个示例中,我们定义了三个任务(job1, job2, job3)并使用 schedule 模块来安排它们每 5 秒,10 秒,15 秒执行一次。然后我们使用 while 循环来持续运行程序,并在每次循环中调用 schedule.run_pending() 来检查是否有任务需要执行。
请注意,schedule模块需要单独安装。
```python
pip install schedule
```
相关问题
python编写定时开关机
在Python中编写定时开关机脚本通常需要借助操作系统提供的功能,比如`os`模块和`schedule`库。这里是一个简单的例子,使用`schedule`库来设置每天的定时任务:
```python
import schedule
import time
from subprocess import call
def job():
# 开机命令
call(["shutdown", "/r", "reboot"], shell=True)
# 关机命令
# call(["shutdown", "/s", "poweroff"], shell=True)
# 如果你想要在特定时间开机
def start_computer(hour, minute):
schedule.every().day.at(f"{hour}:{minute}").do(job)
while True:
schedule.run_pending()
time.sleep(60) # 每分钟检查一次任务
# 如果你想设定关机时间
def shutdown_computer(hour, minute):
schedule.every().day.at(f"{hour}:{minute}").do(lambda: job())
while True:
schedule.run_pending()
time.sleep(60)
# 调用相应函数并传入所需的时间(假设你希望在每天的8:00开机,22:00关机)
start_computer(8, 0)
shutdown_computer(22, 0)
# 运行程序直到用户手动停止
```
请注意,这只是一个基础示例,实际应用可能需要处理异常情况,并且在Windows上使用`shutdown`命令可能会有所不同。此外,这个脚本将一直运行,直到你手动停止它。
python定时开关
您好!感谢您的提问。
要在Python中实现定时开关功能,您可以使用Python的time模块或者第三方库schedule来实现。以下是两种方法的示例代码:
方法一:使用time模块
```python
import time
def switch_on():
print("开关已打开")
def switch_off():
print("开关已关闭")
def timer_switch():
while True:
current_time = time.strftime("%H:%M:%S", time.localtime())
if current_time == "08:00:00":
switch_on()
elif current_time == "18:00:00":
switch_off()
time.sleep(1) # 每隔1秒检查一次时间
timer_switch()
```
上述代码中,使用了time模块的`strftime`方法获取当前时间,并通过比较判断是否到达指定的开关时间。通过循环和`time.sleep`方法实现定时检查。
方法二:使用schedule库
如果您希望更加灵活地设置定时任务,可以使用第三方库schedule。请确保您已经在Python环境中安装了schedule库。
```python
import schedule
import time
def switch_on():
print("开关已打开")
def switch_off():
print("开关已关闭")
def schedule_switch():
schedule.every().day.at("08:00").do(switch_on)
schedule.every().day.at("18:00").do(switch_off)
while True:
schedule.run_pending()
time.sleep(1) # 每隔1秒检查一次任务
schedule_switch()
```
上述代码中,使用了schedule库来设置定时任务,通过`schedule.every().day.at("时间").do(函数)`来设置每天的开关时间。通过循环和`schedule.run_pending`方法实现定时检查和执行任务。
希望以上代码能满足您的需求,如果有任何问题,请随时追问!
阅读全文
相关推荐
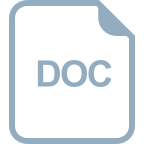
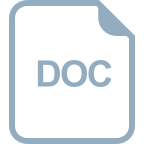
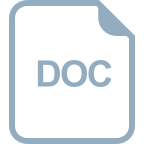
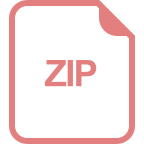

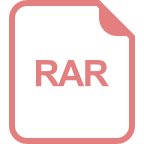
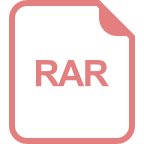
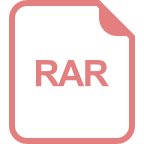
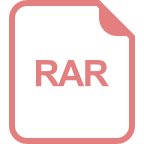
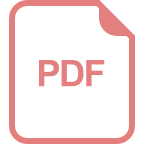
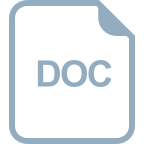
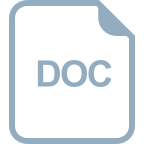
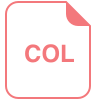
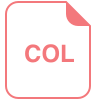


