使用spring cloud搭建微服务项目
时间: 2023-11-03 17:58:14 浏览: 54
搭建微服务项目一般需要以下步骤:
1. 创建父项目
在项目根目录下创建一个父项目,作为所有微服务子项目的父项目。在父项目的pom.xml文件中,配置Spring Cloud和其他依赖的版本号,如下所示:
```
<dependencyManagement>
<dependencies>
<!-- Spring Cloud dependencies -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>2020.0.2</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
```
2. 创建微服务子项目
在父项目下创建多个微服务子项目,每个子项目都是一个独立的服务模块,可以有自己的数据模型、业务逻辑、数据库等。每个子项目都需要配置Spring Boot和Spring Cloud相关依赖,如下所示:
```
<dependencies>
<!-- Spring Boot dependencies -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Cloud dependencies -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
</dependencies>
```
3. 配置微服务
每个微服务都需要有自己的配置文件,例如application.yml或application.properties。在配置文件中,需要指定微服务的端口号、注册中心地址、数据源等信息。
```
server:
port: 8080
spring:
application:
name: user-service
datasource:
url: jdbc:mysql://localhost:3306/user_db
username: root
password: root
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka/
```
4. 注册微服务
微服务需要注册到注册中心,以便其他微服务可以发现它并调用它的接口。可以使用Eureka或Consul等开源组件作为注册中心。在微服务的配置文件中,需要指定注册中心的地址和端口号。
```
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka/
```
5. 调用微服务
微服务之间可以通过HTTP或RPC调用接口进行通信。可以使用Feign或Ribbon等Spring Cloud组件来实现微服务之间的调用。
例如,在一个微服务中调用另一个微服务的接口,可以使用以下代码:
```
@FeignClient(name = "user-service")
public interface UserServiceClient {
@GetMapping("/users/{id}")
User getUserById(@PathVariable("id") Long id);
}
```
以上就是使用Spring Cloud搭建微服务项目的基本步骤。当然,在实际项目中还需要考虑一些其他因素,例如安全性、性能、可伸缩性等。
相关推荐
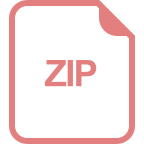
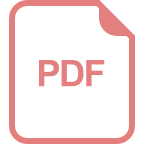














