python发起http请求
时间: 2023-10-16 20:11:47 浏览: 81
在Python中,可以使用内置的`urllib`库,或第三方库`requests`来发起HTTP请求。
以下是使用`urllib`库发起GET请求的示例代码:
```python
import urllib.request
response = urllib.request.urlopen('http://www.example.com')
html = response.read()
print(html)
```
以下是使用`requests`库发起GET请求的示例代码:
```python
import requests
response = requests.get('http://www.example.com')
print(response.text)
```
要发起POST请求,可以使用`requests`库:
```python
import requests
data = {'param1': 'value1', 'param2': 'value2'}
response = requests.post('http://www.example.com', data=data)
print(response.text)
```
需要注意的是,在使用`requests`库时,需要先使用`pip`安装该库,即在命令行中执行:
```
pip install requests
```
相关问题
python发起接口请求
以下是两种Python发起接口请求的例子:
1. 使用requests库发起HTTP请求
```python
import requests
# 请求的url
request_url = 'https://xxxx'
# 请求的参数
param = {
'param1': 'xxxx'
}
# 请求的数据内容,文件类型的数据
fileData = {'media': open('file.txt', 'rb')}
# 发起POST请求
r = requests.post(url=request_url, params=param, files=fileData)
# 输出响应结果
print(r.text)
```
2. 使用telnet发起dubbo请求
```python
import telnetlib
# 连接dubbo服务
tn = telnetlib.Telnet('localhost', 20880)
# 发送dubbo请求
tn.write(b'invoke com.xxx.XxxService.xxxMethod("param1", "param2")\n')
# 获取响应结果
result = tn.read_until(b'\n')
print(result.decode('utf-8'))
# 关闭连接
tn.close()
```
python 发起表单post请求
可以使用Python的requests模块来发起表单POST请求,示例代码如下:
```python
import requests
url = 'http://httpbin.org/post' # POST请求的URL
data = {'key1': 'value1', 'key2': 'value2'} # POST请求的数据
response = requests.post(url, data=data) # 发起POST请求
print(response.text) # 输出响应内容
```
在上面的示例代码中,首先定义了POST请求的URL和数据,然后使用requests模块的post方法发起请求,并将响应存储在response变量中,最后输出响应内容。如果需要在请求中添加请求头、cookies等信息,可以在post方法中传递相应的参数。
阅读全文
相关推荐
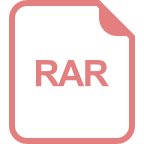
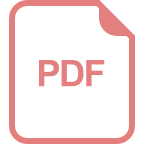
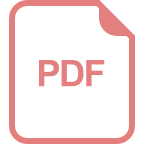
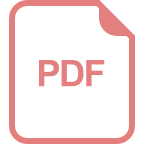
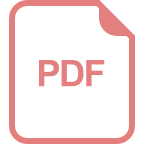
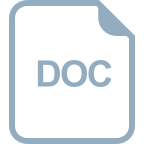
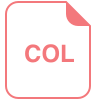





