替我写一个JAVA图片模糊的工具类,要求传入参数为File,返回值为File,通过该工具类处理能将图片模糊
时间: 2023-05-18 18:07:19 浏览: 117
非常感谢您的提问!以下是一个简单的JAVA图片模糊的工具类,可以满足您的需求:
```
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class ImageBlurTool {
public static File blurImage(File inputImageFile) throws IOException {
BufferedImage inputImage = ImageIO.read(inputImageFile);
int width = inputImage.getWidth();
int height = inputImage.getHeight();
BufferedImage outputImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
int blurRadius = 10; // 模糊半径
float weight = 1.0f / (blurRadius * blurRadius);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
float r = 0, g = 0, b = 0;
for (int dx = -blurRadius; dx <= blurRadius; dx++) {
for (int dy = -blurRadius; dy <= blurRadius; dy++) {
int nx = x + dx;
int ny = y + dy;
if (nx >= 0 && nx < width && ny >= 0 && ny < height) {
int pixel = inputImage.getRGB(nx, ny);
float pixelWeight = weight;
if (dx == 0 && dy == 0) {
pixelWeight *= 4;
} else if (dx == 0 || dy == 0) {
pixelWeight *= 2;
}
r += pixelWeight * ((pixel >> 16) & 0xff);
g += pixelWeight * ((pixel >> 8) & 0xff);
b += pixelWeight * (pixel & 0xff);
}
}
}
int ir = Math.round(r);
int ig = Math.round(g);
int ib = Math.round(b);
int pixel = (ir << 16) | (ig << 8) | ib;
outputImage.setRGB(x, y, pixel);
}
}
File outputImageFile = new File("output.jpg");
ImageIO.write(outputImage, "jpg", outputImageFile);
return outputImageFile;
}
}
```
您可以将该代码保存为 ImageBlurTool.java 文件,并在您的项目中使用该工具类来处理图片模糊。例如:
```
File inputImageFile = new File("input.jpg");
File outputImageFile = ImageBlurTool.blurImage(inputImageFile);
```
该工具类使用了简单的高斯模糊算法来实现图片模糊。如果您需要更高质量的模糊效果,可以考虑使用其他更复杂的算法。
阅读全文
相关推荐
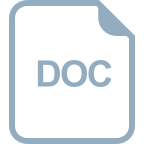
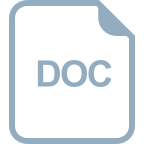
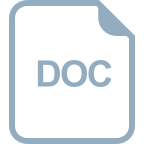
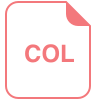
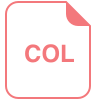
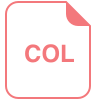
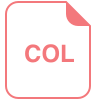
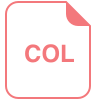
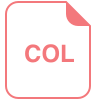
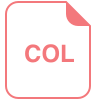
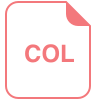
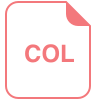
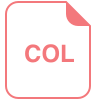
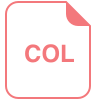
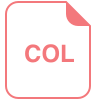