使用pthon将图片裁剪成指定正方形大小
时间: 2023-06-04 19:04:40 浏览: 216
可以使用PIL库来实现这个功能。具体步骤如下:
1. 使用PIL库打开图片:`img = Image.open('path/to/image.jpg')`
2. 获取原始图片的宽和高:`width, height = img.size`
3. 计算要裁剪的区域:如果宽大于高,裁剪出中心部分的正方形区域;如果高大于宽,同样裁剪出中心部分的正方形区域。具体代码如下:
```
if width > height:
left = (width - height) / 2
right = (width + height) / 2
top = 0
bottom = height
else:
left = 0
right = width
top = (height - width) / 2
bottom = (height + width) / 2
region = img.crop((left, top, right, bottom))
```
4. 将裁剪后的区域重新调整为指定大小:`square_img = region.resize((size, size), Image.ANTIALIAS)`
5. 保存新图片:`square_img.save('path/to/new_image.jpg')`
相关问题
请为我提供代码将图片按照指定大小裁剪成正方形
好的,以下是一段 Python 代码可以将图片按照指定大小裁剪成正方形:
```python
from PIL import Image
def crop_to_square(image_path, size):
with Image.open(image_path) as image:
width, height = image.size
new_size = min(width, height)
left = (width - new_size) // 2
top = (height - new_size) // 2
right = (width + new_size) // 2
bottom = (height + new_size) // 2
image = image.crop((left, top, right, bottom))
image = image.resize((size, size))
return image
# example usage
square_image = crop_to_square("path/to/image.jpg", 256)
square_image.save("path/to/square_image.jpg")
```
请注意,上述代码依赖于 `Pillow` 库,你需要首先安装 `Pillow` 才能运行该代码。
阅读全文
相关推荐
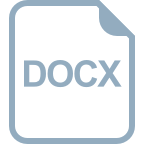
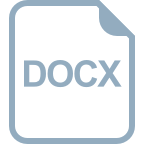
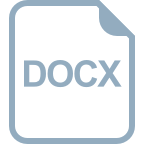
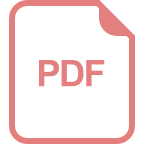
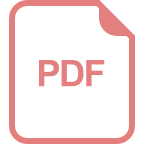
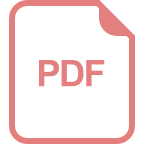
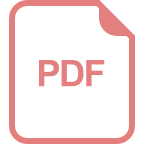
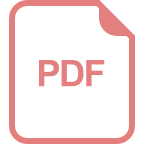
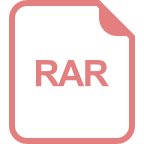
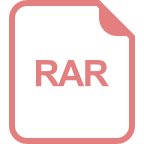
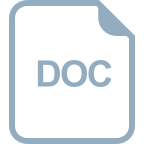
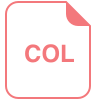
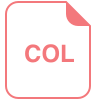
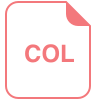
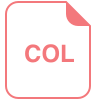