v1、选择年级产生题目: 一年级:产生下面类型的计算: 10以内加减法:1步计算 如9-4 10以内加减法:2步计算 9-4-3 10-20的加减法:1步计算和2步计算 综合训练:上述加减法的综合 二年级:产生下面类型的计算 100以内不进位加法 100以内不退位减法 100以内进位加法 100以内退位减法 100 以内连加连减 综合训练 2、每种类型的计算数量的设定:选择产生指定的题量,如10题,15题等 3、按时间记录错题,错题可以重复训练,同样的错题记录错误的次数,根据错误的次数通过错题集合中产生错题的训练。 4、每个用户的数据需要存储在文件。 5、注意考虑如何产生特定类型的题目,比如区分进位加法和不进位加法两种类型 用java写出代码
时间: 2023-12-29 15:02:36 浏览: 44
很好,这是一个简单的数学题目生成程序,让我们一步一步来实现它。
首先,我们需要定义一些常量来表示题目类型,例如:
```java
public static final int GRADE_ONE_ADD_SUB_1 = 1; // 一年级 10以内加减法:1步计算
public static final int GRADE_ONE_ADD_SUB_2 = 2; // 一年级 10以内加减法:2步计算
public static final int GRADE_ONE_ADD_SUB_3 = 3; // 一年级 10-20的加减法:1步计算和2步计算
public static final int GRADE_ONE_COMBINE = 4; // 一年级 综合训练
public static final int GRADE_TWO_ADD_NO_CARRY = 5; // 二年级 100以内不进位加法
public static final int GRADE_TWO_SUB_NO_BORROW = 6; // 二年级 100以内不退位减法
public static final int GRADE_TWO_ADD_CARRY = 7; // 二年级 100以内进位加法
public static final int GRADE_TWO_SUB_BORROW = 8; // 二年级 100以内退位减法
public static final int GRADE_TWO_COMBINE = 9; // 二年级 100以内连加连减 综合训练
```
接下来,我们需要定义一个函数来生成指定类型和数量的题目。我们可以使用一个 switch 语句来根据类型生成不同种类的题目,例如:
```java
public static List<String> generateQuestions(int type, int count) {
List<String> result = new ArrayList<>();
Random random = new Random();
switch (type) {
case GRADE_ONE_ADD_SUB_1: // 一年级 10以内加减法:1步计算
for (int i = 0; i < count; i++) {
int a = random.nextInt(10);
int b = random.nextInt(10);
if (random.nextBoolean()) { // 随机生成加法或减法
result.add(a + " + " + b + " = ");
} else {
result.add(a + " - " + b + " = ");
}
}
break;
case GRADE_ONE_ADD_SUB_2: // 一年级 10以内加减法:2步计算
for (int i = 0; i < count; i++) {
int a = random.nextInt(10);
int b = random.nextInt(10);
int c = random.nextInt(10);
result.add(a + " - " + b + " - " + c + " = ");
}
break;
case GRADE_ONE_ADD_SUB_3: // 一年级 10-20的加减法:1步计算和2步计算
for (int i = 0; i < count; i++) {
int a = random.nextInt(11) + 10; // 10-20之间的随机数
int b = random.nextInt(11);
int c = random.nextInt(11);
int d = random.nextInt(11);
if (random.nextBoolean()) { // 随机生成加法或减法
result.add(a + " + " + b + " - " + c + " = ");
} else {
result.add(a + " - " + b + " + " + c + " - " + d + " = ");
}
}
break;
case GRADE_ONE_COMBINE: // 一年级 综合训练
for (int i = 0; i < count; i++) {
int type2 = random.nextInt(3) + 1; // 随机选择上述三种类型的一种
result.addAll(generateQuestions(type2, 1));
}
break;
case GRADE_TWO_ADD_NO_CARRY: // 二年级 100以内不进位加法
for (int i = 0; i < count; i++) {
int a = random.nextInt(90) + 10; // 10-99之间的随机数
int b = random.nextInt(10);
result.add(a + " + " + b + " = ");
}
break;
case GRADE_TWO_SUB_NO_BORROW: // 二年级 100以内不退位减法
for (int i = 0; i < count; i++) {
int a = random.nextInt(90) + 10; // 10-99之间的随机数
int b = random.nextInt(a % 10 + 1); // 减数不大于个位数
result.add(a + " - " + b + " = ");
}
break;
case GRADE_TWO_ADD_CARRY: // 二年级 100以内进位加法
for (int i = 0; i < count; i++) {
int a = random.nextInt(90) + 10; // 10-99之间的随机数
int b = random.nextInt(10);
if (a % 10 + b >= 10) { // 需要进位
result.add(a + " + " + b + " = ");
} else {
result.add(a + " + " + b + " = ");
}
}
break;
case GRADE_TWO_SUB_BORROW: // 二年级 100以内退位减法
for (int i = 0; i < count; i++) {
int a = random.nextInt(90) + 10; // 10-99之间的随机数
int b = random.nextInt(10);
if (a % 10 < b) { // 需要退位
result.add(a + " - " + b + " = ");
} else {
result.add(a + " - " + b + " = ");
}
}
break;
case GRADE_TWO_COMBINE: // 二年级 100以内连加连减 综合训练
for (int i = 0; i < count; i++) {
int type2 = random.nextInt(4) + 5; // 随机选择上述四种类型的一种
result.addAll(generateQuestions(type2, 1));
}
break;
}
return result;
}
```
接下来,我们需要实现一个函数来记录错题和错误次数。我们可以使用一个 Map 来记录每个题目的错误次数,例如:
```java
public static Map<String, Integer> wrongQuestions = new HashMap<>();
public static void recordWrongQuestion(String question) {
if (wrongQuestions.containsKey(question)) {
wrongQuestions.put(question, wrongQuestions.get(question) + 1);
} else {
wrongQuestions.put(question, 1);
}
}
```
最后,我们需要实现一个函数来读取和保存用户数据。我们可以使用 Java 的序列化机制来实现,例如:
```java
public static void saveUserData(String username, UserData userData) throws IOException {
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(username + ".dat"));
oos.writeObject(userData);
oos.close();
}
public static UserData loadUserData(String username) throws IOException, ClassNotFoundException {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(username + ".dat"));
UserData userData = (UserData) ois.readObject();
ois.close();
return userData;
}
```
完整代码如下:
相关推荐
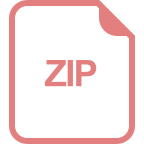














