在上一段代码的基础上3.在已经建立的单链表第i个位置插入一个新元素e,将插入新元素之后的单链表输出。 用C语言
时间: 2023-06-07 13:07:10 浏览: 71
可以使用以下代码来实现单链表的插入操作,并输出插入后的单链表。
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
typedef struct Node Node;
// 在单链表第i个位置插入元素e
void insert(Node** headRef, int i, int e) {
Node* newNode = (Node*) malloc(sizeof(Node));
newNode->data = e;
newNode->next = NULL;
if (i == 1) {
newNode->next = *headRef;
*headRef = newNode;
return;
}
Node* current = *headRef;
for (int j = 1; j < i - 1 && current != NULL; j++) {
current = current->next;
}
if (current == NULL) {
printf("Error: Invalid index");
return;
}
newNode->next = current->next;
current->next = newNode;
}
// 输出单链表
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
Node* head = NULL;
// 在单链表末尾添加元素
for (int i = 1; i <= 5; i++) {
insert(&head, i, i);
}
// 在单链表第3个位置插入元素
insert(&head, 3, 10);
// 输出单链表
printList(head);
return 0;
}
```
以上代码首先定义了一个 `Node` 结构体表示单链表中的每个节点,然后定义了 `insert` 函数实现在单链表中插入新元素,并定义了 `printList` 函数用于输出单链表。
在 `main` 函数中,首先在单链表末尾添加了5个元素(即使 i 从 1 自增到 5,每次插入的元素值都与 i 相同),随后调用 `insert` 函数将元素值为 10 的新节点插入到单链表的第 3 个位置,最后调用 `printList` 函数输出插入后的单链表。
阅读全文
相关推荐
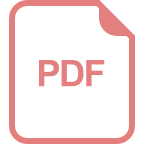
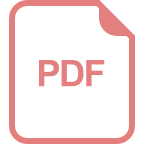
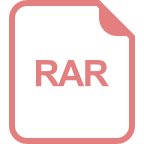















