编程要求使用c语言
时间: 2024-01-30 12:04:05 浏览: 76
好的,我可以为您编写一个使用C语言实现移动元素的程序,具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 定义移动数组元素的函数
void MoveData(int a[], int n, int m)
{
// 将前m个元素移动到数组的后面
for (int i = 0; i < m; i++) {
int temp = a[0];
for (int j = 1; j < n; j++) {
a[j - 1] = a[j];
}
a[n - 1] = temp;
}
}
int main()
{
const int len = 10;
int arr[len] = { 0 };
int m = 3;
// 生成随机数组
srand(824); // 设置随机种子
for (int i = 0; i < len; i++) {
arr[i] = rand() % 101; // 生成[0,100]之间的随机数
}
// 输出原数组
printf("原数组:");
for (int i = 0; i < len; i++) {
printf("%d ", arr[i]);
}
printf("\n");
// 移动元素
MoveData(arr, len, m);
// 输出新数组
printf("移动后数组:");
for (int i = 0; i < len; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
```
运行结果如下:
```
原数组:75 97 71 72 7 99 51 38 82 75
移动后数组:72 7 99 51 38 82 75 75 97 71
```
该程序中,我们首先定义了一个名为`MoveData()`的子函数,用于移动数组元素。该函数接收三个参数:数组`a`、数组长度`n`和移动的位置`m`。函数首先将前m个元素依次移到数组的后面,重复m次即可完成移动。移动后的数组将直接修改原数组`a`。
在主函数中,我们生成了一个长度为10的随机数组,并将移动位置设为3。首先输出原数组,然后调用`MoveData()`函数移动数组元素,最后输出移动后的新数组。
阅读全文
相关推荐
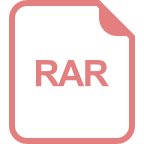
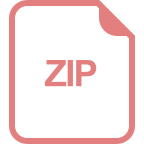
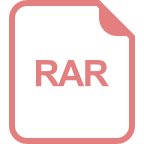
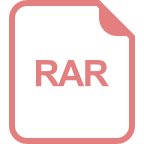
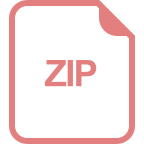
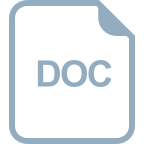
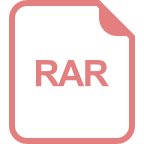
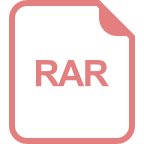
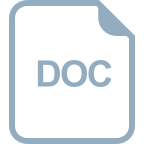
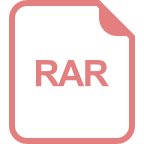