现有文件夹A内有若干张滑坡二进制掩码图像,如何在pycharm软件中利用代码求得值为1的多边形也就是滑坡体的目标检测VOC格式的标签以xml文件(应与每张图片一一对应)形式存贮到文件夹B,注意每张图片内有四个滑坡体,也就是每张照片需要四个检测框(可以通过多边形的连续性判断)
时间: 2024-05-08 08:19:08 浏览: 16
可以使用OpenCV和xml.etree.ElementTree模块实现,代码如下:
```python
import cv2
import numpy as np
import os
import xml.etree.ElementTree as ET
folder_A = "path/to/folder/A"
folder_B = "path/to/folder/B"
# Function to generate VOC format XML file
def generate_xml(filename, width, height, objects):
annotation = ET.Element("annotation")
ET.SubElement(annotation, "folder").text = "Folder name"
ET.SubElement(annotation, "filename").text = filename
ET.SubElement(annotation, "path").text = os.path.join(os.getcwd(), filename)
size = ET.SubElement(annotation, "size")
ET.SubElement(size, "width").text = str(width)
ET.SubElement(size, "height").text = str(height)
ET.SubElement(size, "depth").text = "3"
for obj in objects:
ob = ET.SubElement(annotation, "object")
ET.SubElement(ob, "name").text = "slip"
ET.SubElement(ob, "pose").text = "Unspecified"
ET.SubElement(ob, "truncated").text = "0"
ET.SubElement(ob, "difficult").text = "0"
bbox = ET.SubElement(ob, "bndbox")
ET.SubElement(bbox, "xmin").text = str(obj[0])
ET.SubElement(bbox, "ymin").text = str(obj[1])
ET.SubElement(bbox, "xmax").text = str(obj[2])
ET.SubElement(bbox, "ymax").text = str(obj[3])
xml_str = ET.tostring(annotation)
return xml_str
# Loop through all images in folder A
for file in os.listdir(folder_A):
if file.endswith(".bin"):
# Read binary image
img_bin = np.fromfile(os.path.join(folder_A, file), np.uint8)
img = cv2.imdecode(img_bin, cv2.IMREAD_GRAYSCALE)
# Convert binary to binary edge image
ret, thresh = cv2.threshold(img, 127, 255, cv2.THRESH_BINARY)
edges = cv2.Canny(thresh, 100, 200)
# Find contours and draw bounding boxes
contours, hierarchy = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
objects = []
for cnt in contours:
# Approximate contour as polygon
epsilon = 0.01 * cv2.arcLength(cnt, True)
approx = cv2.approxPolyDP(cnt, epsilon, True)
# Check if polygon has 4 sides (i.e., a rectangle)
if len(approx) == 4:
x, y, w, h = cv2.boundingRect(approx)
objects.append([x, y, x + w, y + h])
# Generate XML file
xml_str = generate_xml(file + ".xml", img.shape[1], img.shape[0], objects)
# Save XML file
with open(os.path.join(folder_B, file + ".xml"), "wb") as f:
f.write(xml_str)
```
需要安装OpenCV和numpy模块,代码中的文件夹路径需要根据实际情况修改。
相关推荐
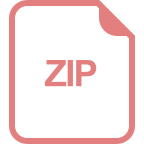
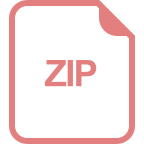
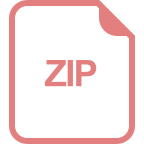













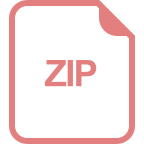
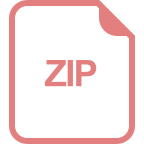