使用支付宝沙箱生成收款码代码实现
时间: 2024-03-01 12:43:12 浏览: 28
好的,以下是使用支付宝沙箱生成收款码的代码实现:
``` python
import requests
# 支付宝沙箱接口地址
url = "https://openapi.alipaydev.com/gateway.do"
# 支付宝沙箱应用的APPID
app_id = "你的APPID"
# 支付宝沙箱应用的私钥
app_private_key = """
-----BEGIN RSA PRIVATE KEY-----
你的私钥
-----END RSA PRIVATE KEY-----
"""
# 支付宝沙箱应用的公钥
alipay_public_key = """
-----BEGIN PUBLIC KEY-----
支付宝公钥
-----END PUBLIC KEY-----
"""
# 构建请求参数
params = {
"app_id": app_id,
"method": "alipay.marketing.cashlessvoucher.template.create",
"format": "JSON",
"charset": "utf-8",
"sign_type": "RSA2",
"timestamp": "2022-01-01 00:00:00",
"version": "1.0",
"biz_content": {
"template_name": "测试收款码",
"voucher_type": "cashless",
"brand_name": "测试品牌",
"voucher_desc": "测试描述",
"publish_start_time": "2022-01-01 00:00:00",
"publish_end_time": "2023-01-01 00:00:00",
"use_rule": {
"use_condition": {
"suit_shops": {
"shop_id": [
"001",
"002",
"003"
]
}
}
}
}
}
# 生成签名
from urllib.parse import quote_plus
from hashlib import sha256
from base64 import encodebytes
from Crypto.PublicKey import RSA
from Crypto.Signature import PKCS1_v1_5
sign_params = "&".join("{}={}".format(k, quote_plus(str(v))) for k, v in sorted(params.items()))
sign_string = "&".join(["POST", quote_plus("/gateway.do"), quote_plus(sign_params)])
sign_key = RSA.import_key(app_private_key)
signer = PKCS1_v1_5.new(sign_key)
signature = encodebytes(signer.sign(sha256(sign_string.encode("utf-8")))).decode("utf-8").replace("\n", "")
# 发送请求
headers = {
"Content-Type": "application/json;charset=utf-8",
}
data = {
**params,
"sign": signature,
}
response = requests.post(url, headers=headers, json=data)
# 解析响应
import json
response_data = json.loads(response.content.decode("utf-8"))
qr_code = response_data["alipay_cashless_voucher_template_create_response"]["qr_code"]
print(qr_code)
```
在上面的代码中,我们通过 `alipay.marketing.cashlessvoucher.template.create` 接口创建了一个无需现金支付的优惠券模板,并生成了一个收款码。其中,我们需要将支付宝沙箱应用的APPID、私钥和公钥替换成自己的。
成功执行后,会输出生成的收款码。
相关推荐
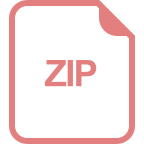














