写一个算法,将两个带头结点的单链表LA和LB合并为一个单循环链表,其头指针为LA。
时间: 2024-10-29 22:15:21 浏览: 58
为了将两个单链表LA和LB合并为一个单循环链表,我们可以按照以下步骤编写算法:
1. **创建新链表**:
- 创建一个新的链表LC,将其头节点初始化为LA的头节点。
2. **合并链表**:
- 遍历LA和LB,分别从两个链表的头节点开始。
- 当遍历到其中一个链表结束时,将另一个链表剩余部分接到LC的尾部,并将最后一个节点的下一个节点指向头节点形成循环。
3. **连接节点**:
- 如果LA还有节点:
- 将LA当前节点的next指向前一个节点(即LC的尾节点)。
- 如果LB还有节点:
- 将LB当前节点的next指向前一个节点(同样,这里是LC的尾节点)。
4. **返回合并后的链表**:
- 最终LC就是合并后的单循环链表,其头节点依然是LA的头节点。
以下是这个算法的一个伪代码示例:
```python
def merge_lists(LA, LB):
LC = LA.head # 新链表的头节点
tail = LA.head
while LA.current is not None and LB.current is not None:
if LA.current.value < LB.current.value:
tail.next = LA.current
LA.current = LA.current.next
else:
tail.next = LB.current
LB.current = LB.current.next
tail = tail.next
# 将剩余的链表接到LC尾部
if LA.current is not None:
tail.next = LA.current
LA.current.next = LA.head
elif LB.current is not None:
tail.next = LB.current
LB.current.next = LB.head
return LA.head
```
阅读全文
相关推荐
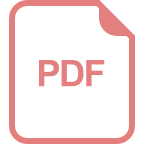
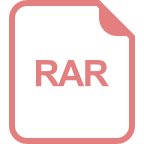
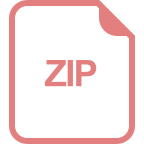
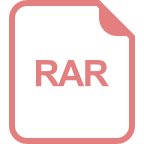
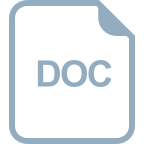
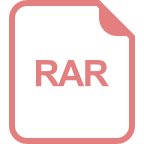
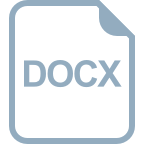
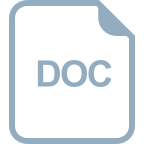
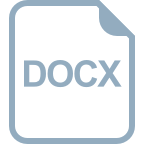
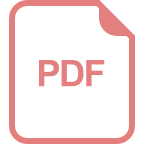
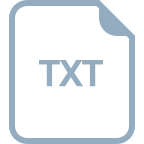
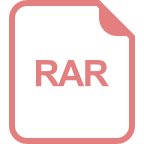
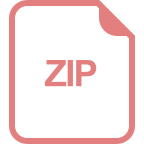
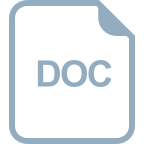
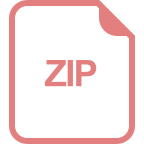
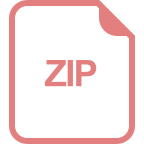
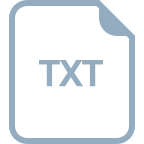