首页Nodejs ORM框架写SqlServer的数据库的连接
Nodejs ORM框架写SqlServer的数据库的连接
时间: 2024-05-22 13:14:22 浏览: 21
方式有哪些?
可以使用以下几种方式连接SqlServer数据库:
1.使用Node.js的mssql模块,直接连接SqlServer数据库
2.使用Sequelize ORM框架,支持SqlServer数据库
3.使用TypeORM ORM框架,支持SqlServer数据库
4.使用Knex.js查询构建器,支持SqlServer数据库。
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
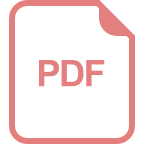
nodejs基于mssql模块连接sqlserver数据库的简单封装操作示例
主要介绍了nodejs基于mssql模块连接sqlserver数据库的简单封装操作,结合实例形式分析了nodejs中mssql模块的安装与操作sqlserver数据库相关使用技巧,需要的朋友可以参考下
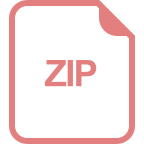
校园网Web平台二手商品交易系统的设计与实现研究论文
python有趣的库本系统是一款基于JSP/J2EE技术的校园网二手交易平台,采用Java语言开发。它采用流行的B/S架构,以互联网为基础运行,服务端安装简便,客户端则只需联网即可通过浏览器轻松访问。无需复杂的C/S模式安装、配置和维护流程。系统利用Java的面向对象、跨平台、高安全、高稳定、多线程等特性,结合其对网络编程技术的支持,使得本平台具有极高的实用价值。
系统结构清晰,分为三大核心部分:JavaBeans负责业务逻辑处理,JSP结合HTML和JavaScript负责界面展示,Servlet则作为中间件,并通过JDBC-ODBC桥接器与SQL Server 2000数据库进行交互,确保数据访问的高效和稳定。。内容来源于网络分享,如有侵权请联系我删除。另外如果没有积分的同学需要下载,请私信我。
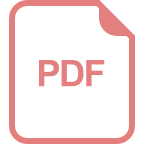
京瓷TASKalfa系列维修手册:安全与操作指南
"该资源是一份针对京瓷TASKalfa系列多款型号打印机的维修手册,包括TASKalfa 2020/2021/2057,TASKalfa 2220/2221,TASKalfa 2320/2321/2358,以及DP-480,DU-480,PF-480等设备。手册标注为机密,仅供授权的京瓷工程师使用,强调不得泄露内容。手册内包含了重要的安全注意事项,提醒维修人员在处理电池时要防止爆炸风险,并且应按照当地法规处理废旧电池。此外,手册还详细区分了不同型号产品的打印速度,如TASKalfa 2020/2021/2057的打印速度为20张/分钟,其他型号则分别对应不同的打印速度。手册还包括修订记录,以确保信息的最新和准确性。"
本文档详尽阐述了京瓷TASKalfa系列多功能一体机的维修指南,适用于多种型号,包括速度各异的打印设备。手册中的安全警告部分尤为重要,旨在保护维修人员、用户以及设备的安全。维修人员在操作前必须熟知这些警告,以避免潜在的危险,如不当更换电池可能导致的爆炸风险。同时,手册还强调了废旧电池的合法和安全处理方法,提醒维修人员遵守地方固体废弃物法规。
手册的结构清晰,有专门的修订记录,这表明手册会随着设备的更新和技术的改进不断得到完善。维修人员可以依靠这份手册获取最新的维修信息和操作指南,确保设备的正常运行和维护。
此外,手册中对不同型号的打印速度进行了明确的区分,这对于诊断问题和优化设备性能至关重要。例如,TASKalfa 2020/2021/2057系列的打印速度为20张/分钟,而TASKalfa 2220/2221和2320/2321/2358系列则分别具有稍快的打印速率。这些信息对于识别设备性能差异和优化工作流程非常有用。
总体而言,这份维修手册是京瓷TASKalfa系列设备维修保养的重要参考资料,不仅提供了详细的操作指导,还强调了安全性和合规性,对于授权的维修工程师来说是不可或缺的工具。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
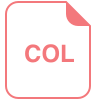
【进阶】入侵检测系统简介
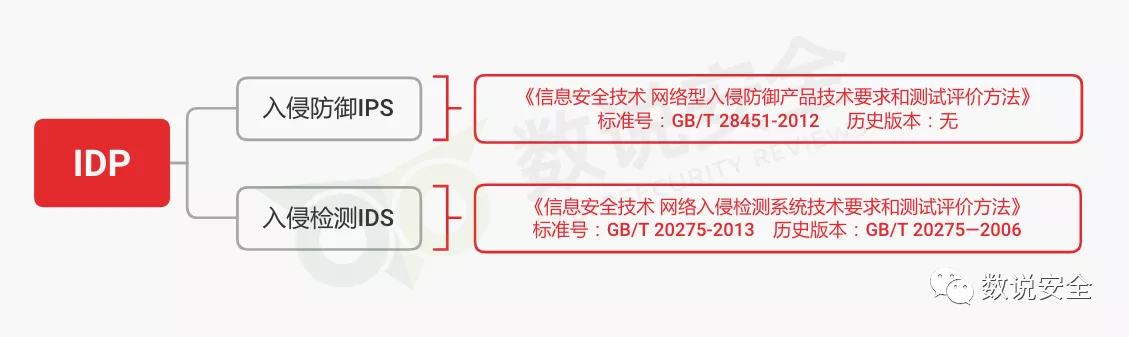
# 1. 入侵检测系统概述**
入侵检测系统(IDS)是一种网络安全工具,用于检测和预防未经授权的访问、滥用、异常或违反安全策略的行为。IDS通过监控网络流量、系统日志和系统活动来识别潜在的威胁,并向管理员发出警报。
IDS可以分为两大类:基于网络的IDS(NIDS)和基于主机的IDS(HIDS)。NIDS监控网络流量,而HIDS监控单个主机的活动。IDS通常使用签名检测、异常检测和行

轨道障碍物智能识别系统开发
轨道障碍物智能识别系统是一种结合了计算机视觉、人工智能和机器学习技术的系统,主要用于监控和管理铁路、航空或航天器的运行安全。它的主要任务是实时检测和分析轨道上的潜在障碍物,如行人、车辆、物体碎片等,以防止这些障碍物对飞行或行驶路径造成威胁。
开发这样的系统主要包括以下几个步骤:
1. **数据收集**:使用高分辨率摄像头、雷达或激光雷达等设备获取轨道周围的实时视频或数据。
2. **图像处理**:对收集到的图像进行预处理,包括去噪、增强和分割,以便更好地提取有用信息。
3. **特征提取**:利用深度学习模型(如卷积神经网络)提取障碍物的特征,如形状、颜色和运动模式。
4. **目标
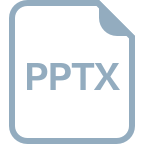
小波变换在视频压缩中的应用
"多媒体通信技术视频信息压缩与处理(共17张PPT).pptx"
多媒体通信技术涉及的关键领域之一是视频信息压缩与处理,这在现代数字化社会中至关重要,尤其是在传输和存储大量视频数据时。本资料通过17张PPT详细介绍了这一主题,特别是聚焦于小波变换编码和分形编码两种新型的图像压缩技术。
4.5.1 小波变换编码是针对宽带图像数据压缩的一种高效方法。与离散余弦变换(DCT)相比,小波变换能够更好地适应具有复杂结构和高频细节的图像。DCT对于窄带图像信号效果良好,其变换系数主要集中在低频部分,但对于宽带图像,DCT的系数矩阵中的非零系数分布较广,压缩效率相对较低。小波变换则允许在频率上自由伸缩,能够更精确地捕捉图像的局部特征,因此在压缩宽带图像时表现出更高的效率。
小波变换与傅里叶变换有本质的区别。傅里叶变换依赖于一组固定频率的正弦波来表示信号,而小波分析则是通过母小波的不同移位和缩放来表示信号,这种方法对非平稳和局部特征的信号描述更为精确。小波变换的优势在于同时提供了时间和频率域的局部信息,而傅里叶变换只提供频率域信息,却丢失了时间信息的局部化。
在实际应用中,小波变换常常采用八带分解等子带编码方法,将低频部分细化,高频部分则根据需要进行不同程度的分解,以此达到理想的压缩效果。通过改变小波的平移和缩放,可以获取不同分辨率的图像,从而实现按需的图像质量与压缩率的平衡。
4.5.2 分形编码是另一种有效的图像压缩技术,特别适用于处理不规则和自相似的图像特征。分形理论源自自然界的复杂形态,如山脉、云彩和生物组织,它们在不同尺度上表现出相似的结构。通过分形编码,可以将这些复杂的形状和纹理用较少的数据来表示,从而实现高压缩比。分形编码利用了图像中的分形特性,将其转化为分形块,然后进行编码,这在处理具有丰富细节和不规则边缘的图像时尤其有效。
小波变换和分形编码都是多媒体通信技术中视频信息压缩的重要手段,它们分别以不同的方式处理图像数据,旨在减少存储和传输的需求,同时保持图像的质量。这两种技术在现代图像处理、视频编码标准(如JPEG2000)中都有广泛应用。

"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依
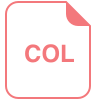
【进阶】Python高级加密库cryptography
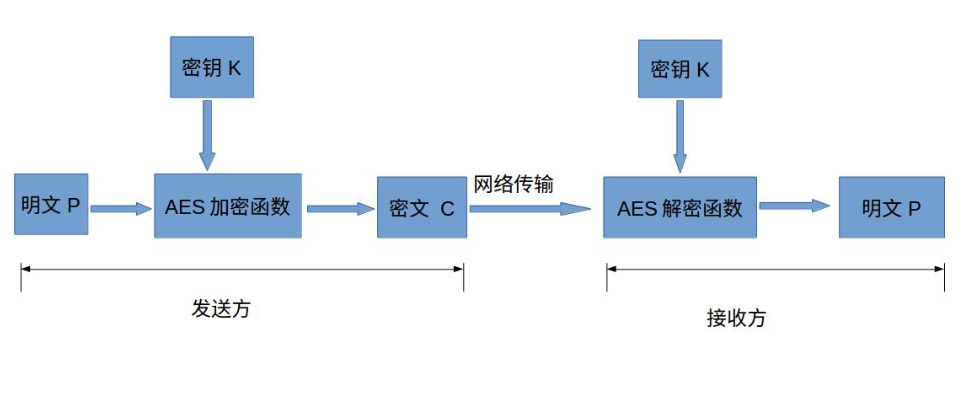
# 2.1 AES加密算法
### 2.1.1 AES加密原理
AES(高级加密标准)是一种对称块密码,由美国国家标准与技术研究院(NIST)于2001年发布。它是一种分组密码,这意味着它一次处理固定大小的数据块(通常为128位)。AES使用密钥长度为128、192或256位的迭代密码,称为Rijndael密码。
Rijndael密码基于以下基本操作:
- 字节替换:将每个字节替换为S盒中的另一个字节。
- 行移位:将每一行

linuxjar包启动脚本
Linux中的jar包通常指的是Java Archive(Java归档文件),它是一个包含Java类、资源和其他相关文件的压缩文件。启动一个Java应用的jar包通常涉及到使用Java的Runtime或JVM(Java虚拟机)。
一个简单的Linux启动jar包的脚本(例如用bash编写)可能会类似于这样:
```bash
#!/bin/bash
# Java启动脚本
# 设置JAVA_HOME环境变量,指向Java安装路径
export JAVA_HOME=/path/to/your/java/jdk
# jar包的路径
JAR_FILE=/path/to/your/applicat