手机验证码请求发送实现
时间: 2024-01-04 12:10:57 浏览: 27
手机验证码请求发送一般需要使用短信服务商提供的 API 接口来实现。以下是一个简单的示例,使用阿里云短信服务的 API 发送验证码:
```python
import requests
import json
# 阿里云短信服务的请求地址和密钥
url = "https://dysmsapi.aliyuncs.com/"
accessKeyId = "your_accessKeyId"
accessSecret = "your_accessSecret"
# 手机号码
phone_number = "your_phone_number"
# 生成的验证码
verification_code = "123456"
# 构造 API 请求参数
data = {
"Action": "SendSms",
"SignName": "your_sign_name",
"TemplateCode": "your_template_code",
"PhoneNumbers": phone_number,
"TemplateParam": json.dumps({"code": verification_code})
}
headers = {
"Content-Type": "application/x-www-form-urlencoded",
"Accept": "application/json",
}
# 发送 API 请求
response = requests.post(url, headers=headers, data=data, auth=(accessKeyId, accessSecret))
# 处理响应结果
if response.status_code == 200:
result = json.loads(response.text)
if result["Code"] == "OK":
print("验证码发送成功")
else:
print("验证码发送失败:", result["Message"])
else:
print("API 请求失败:", response.status_code)
```
其中,需要替换的参数包括:
- `accessKeyId` 和 `accessSecret`:阿里云短信服务的密钥,需要在阿里云控制台中申请并生成。
- `phone_number`:接收验证码的手机号码。
- `verification_code`:生成的验证码。
- `SignName`:短信签名,需要在阿里云控制台中申请并审核通过。
- `TemplateCode`:短信模板代码,需要在阿里云控制台中申请并审核通过。
- `TemplateParam`:短信模板中的参数,需要根据实际模板内容进行替换。
注意:以上示例仅供参考,具体实现方式可能因短信服务商的不同而有所差异。
相关推荐
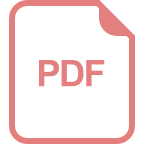
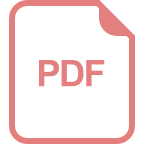














