C语言3.编写一程序,将两个字符串连接起来,结果取代第一个字符串。 (1)用字符数组,不用strcat函数(即自己写一个具有strcat函数功能的函数); (2)用标准库中的strcat函数。
时间: 2024-12-16 21:27:31 浏览: 19
(1)使用字符数组连接字符串(自定义 strcat 功能的函数):
```c
#include <stdio.h>
#include <string.h>
// 自定义 str_concat 函数
void str_concat(char* dest, const char* src) {
int i = strlen(dest); // 获取dest字符串长度
while (dest[i] != '\0') { // 避免越界
i++;
}
for (; *src != '\0'; src++) { // 将源字符串逐个字符复制到dest
dest[i] = *src;
i++;
}
dest[i] = '\0'; // 添加终止符
}
int main() {
char str1[50], str2[] = " appended";
printf("请输入第一个字符串: ");
fgets(str1, sizeof(str1), stdin);
str1[strlen(str1)-1] = '\0'; // 去除换行符
str_concat(str1, str2);
printf("合并后的字符串: %s\n", str1);
return 0;
}
相关问题
c语言编写程序实现两个字符串相连接,不用strcat函数
,该怎么做?
可以通过以下步骤实现两个字符串相连接:
1. 创建一个新的字符数组,用于存储连接后的字符串。
2. 使用循环将第一个字符串中的字符逐个复制到新的字符数组中。
3. 使用另一个循环将第二个字符串中的字符逐个复制到新的字符数组中,从第一个字符串的末尾开始。
4. 在新的字符数组的末尾添加一个空字符 '\0',以表示字符串的结束。
以下是可以实现上述步骤的C语言代码示例:
```c
#include <stdio.h>
void stringConcat(char str1[], char str2[], char newStr[])
{
int i, j;
// 复制第一个字符串
for(i=0; str1[i]!='\0'; i++)
{
newStr[i] = str1[i];
}
// 复制第二个字符串
for(j=0; str2[j]!='\0'; j++)
{
newStr[i+j] = str2[j];
}
// 添加结束符
newStr[i+j] = '\0';
}
int main()
{
char str1[50] = "Hello";
char str2[25] = "World";
char newStr[75];
// 连接两个字符串
stringConcat(str1, str2, newStr);
printf("连接后的字符串为: %s", newStr);
return 0;
}
```
请注意,上述代码仅适用于两个字符串的连接。如果需要连接多个字符串,则需要进行更复杂的操作。
编写一个程序,输入两个字符串并将其连接起来,不要用strcat函数。用C语言代码编写
好的,以下是示例代码:
```
#include <stdio.h>
int main() {
char str1[100], str2[100], result[200];
int i = 0, j = 0;
printf("请输入第一个字符串:");
scanf("%s", str1);
printf("请输入第二个字符串:");
scanf("%s", str2);
// 拷贝第一个字符串到结果数组中
while (str1[i] != '\0') {
result[i] = str1[i];
i++;
}
// 拷贝第二个字符串到结果数组中
while (str2[j] != '\0') {
result[i] = str2[j];
i++;
j++;
}
// 结果数组末尾加上结束符
result[i] = '\0';
printf("连接后的字符串为:%s\n", result);
return 0;
}
```
以上代码中,我们使用了两个 while 循环将两个字符串拷贝到结果数组中,并且在末尾加上了结束符 '\0',最终输出结果即为连接后的字符串。
阅读全文
相关推荐
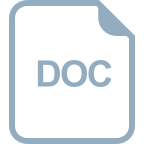
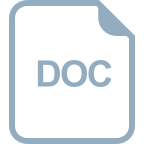
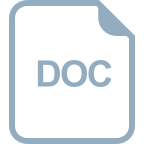
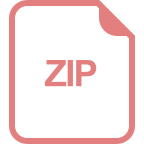
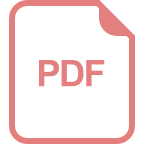
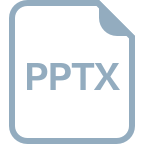
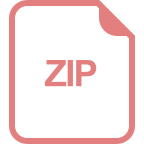
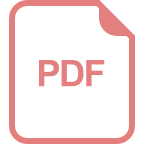
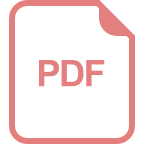
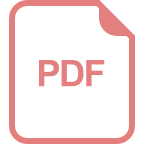
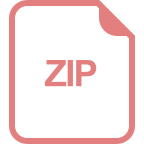
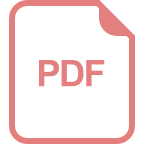
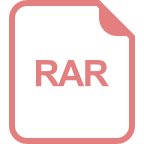
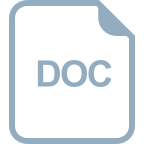
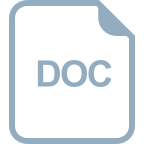