springboot Jpa 如何实现json请求
时间: 2024-11-22 09:47:53 浏览: 15
Spring Boot结合JPA (Java Persistence API) 使用起来非常方便,可以轻松处理JSON请求并映射到数据库操作。以下是实现步骤:
1. **配置Spring Data JPA**:
在`application.properties`或`application.yml`文件中添加JPA的相关配置,如数据源、hibernate属性等。
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
```
2. **创建实体类**:
创建代表数据库表的Java实体类,例如User.java。
```java
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// ...其他字段及getter/setter
}
```
3. **定义Repository接口**:
使用JpaRepository接口或自定义接口来执行CRUD操作,并注入EntityManager。
```java
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
```
4. **控制器类**:
接受JSON请求并在Controller中注入Repository,使用`@RequestBody`注解接收JSON数据。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@Autowired
private UserRepository userRepository;
@PostMapping("/users")
public User createUser(@RequestBody User user) {
return userRepository.save(user);
}
// 其他HTTP方法,比如更新或删除,类似地处理JSON请求
}
```
5. **发送JSON请求**:
使用支持JSON的库(如Jackson),客户端通过HTTP POST向`/users`端点发送包含用户数据的JSON对象。
```javascript
{
"name": "John Doe",
"email": "john.doe@example.com"
}
```
阅读全文
相关推荐
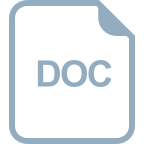
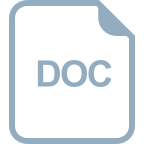
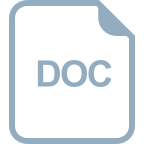
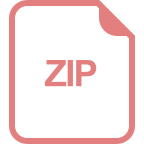
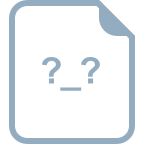
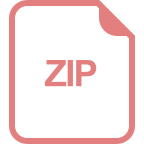
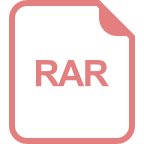
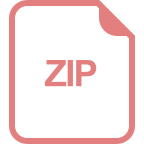
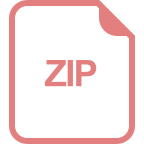
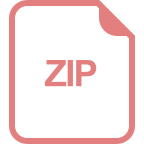
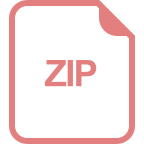
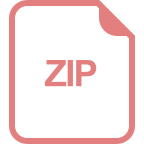
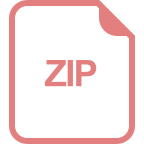
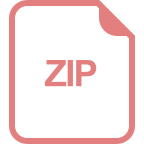

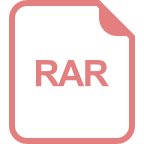
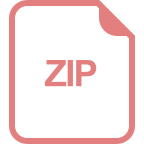
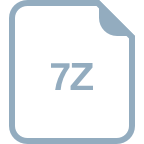
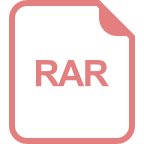