C# 字符串尾部匹配某个字符串
时间: 2024-10-22 14:03:47 浏览: 13
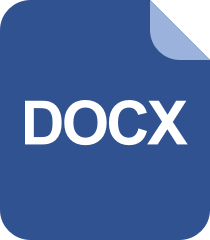
C#操作字符串方法总结
在C#中,如果你想检查一个字符串是否以特定的尾部字符串结束,可以使用`string.EndsWith()`方法。这个方法接收两个参数:一个是你要查找的尾部字符串,另一个是可选的比较标志,用于指定字符编码和区分大小写的行为。
语法如下:
```csharp
bool endsWith = originalString.EndsWith(searchString[, comparison]);
```
- `originalString` 是你要检查的原始字符串。
- `searchString` 是你要寻找的尾部字符串。
- `comparison` 是可选的,它可以是 `StringComparison.CurrentCulture`, `StringComparison.OrdinalIgnoreCase`, 或者其他的枚举值。默认值是 `StringComparison.Ordinal`,即忽略文化因素并区分大小写。
示例:
```csharp
string myString = "Hello, World!";
if (myString.EndsWith("World!")) {
Console.WriteLine("The string ends with 'World!'");
}
```
阅读全文
相关推荐
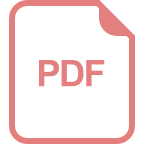
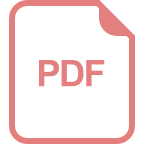


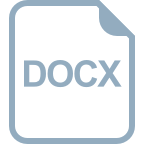
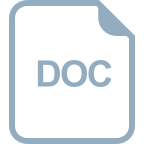
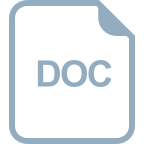
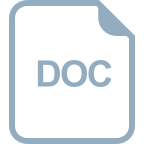
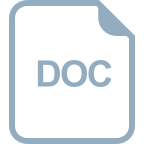





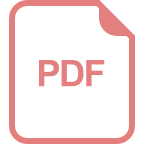
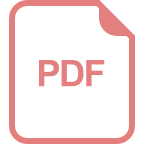
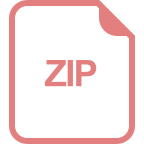