与c++编写程序,输入若干整数到一维数组中,以回车结束,求数组元素的最大值。数组元素个数不超过100。
时间: 2024-10-06 14:01:25 浏览: 50
在C++中,你可以通过以下步骤编写一个简单的程序来实现这个功能:
1. 首先,你需要定义一个一维动态数组来存储整数。由于数组大小有限制,可以使用`std::vector<int>`,它能自动调整大小以适应输入的元素。
```cpp
#include <iostream>
#include <vector>
int main() {
```
2. 接着创建一个空的`vector`,并提示用户输入整数,直到遇到换行符为止。
```cpp
std::vector<int> numbers;
int input;
std::cout << "请输入整数(以回车结束),最多100个:";
while (std::cin >> input && numbers.size() < 100) { // 当输入有效且未达到100个时
numbers.push_back(input); // 将输入添加到数组中
}
```
3. 使用`*begin()`或`begin()`获取数组的第一个元素,然后遍历数组找出最大值。这里假设`*numbers.rbegin()`会返回最大的元素,因为向后迭代可以找到最后一个元素。
```cpp
if (!numbers.empty()) { // 检查数组是否为空
auto max_value = *numbers.rbegin(); // 获取最大值
std::cout << "数组的最大值是:" << max_value << std::endl;
} else {
std::cout << "输入的数组为空。" << std::endl;
}
return 0;
}
```
4. 最后,记得处理输入错误,并确保在循环结束后清理完毕。
```cpp
catch (const std::exception& e) {
std::cerr << "输入错误: " << e.what() << std::endl;
}
return 0;
```
阅读全文
相关推荐
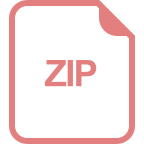
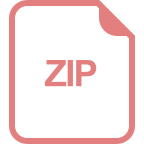
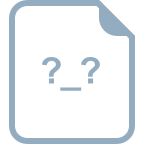















