vue2自定义指令实现图片懒加载
时间: 2023-09-12 11:12:27 浏览: 67
要实现图片懒加载的自定义指令,你可以按照以下步骤进行:
1. 创建一个自定义指令文件,比如 `lazyload.js`。
2. 在 `lazyload.js` 文件中,定义一个全局自定义指令 `v-lazyload`,并添加相应的逻辑。
```javascript
// lazyload.js
// 判断元素是否在可视区域内
function isInViewport(el) {
const rect = el.getBoundingClientRect();
return (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) &&
rect.right <= (window.innerWidth || document.documentElement.clientWidth)
);
}
// 加载图片
function loadImage(el) {
if (el.getAttribute('data-src')) {
el.src = el.getAttribute('data-src');
el.removeAttribute('data-src');
}
}
// Vue 自定义指令
Vue.directive('lazyload', {
inserted: function(el) {
// 初始状态先将图片设置为占位图
el.src = 'placeholder.jpg';
// 判断图片是否在可视区域内,如果在则加载图片
if (isInViewport(el)) {
loadImage(el);
}
},
bind: function(el) {
if (isInViewport(el)) {
loadImage(el);
}
},
scroll: function(el) {
if (isInViewport(el)) {
loadImage(el);
}
}
});
```
3. 在主文件中引入自定义指令文件。
```javascript
// main.js
import Vue from 'vue';
import './lazyload.js'; // 引入自定义指令文件
new Vue({
// ...
}).$mount('#app');
```
4. 在需要懒加载的图片上使用自定义指令 `v-lazyload`。
```html
<template>
<div>
<img v-lazyload data-src="image.jpg" alt="Lazy loaded image">
</div>
</template>
```
这样,当图片进入可视区域时,即可加载对应的图片。记得替换 `placeholder.jpg` 和 `image.jpg` 为你自己的图片路径。
相关推荐
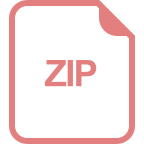
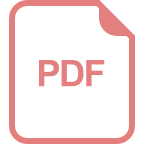













