Overriding method should call `super.onRequestPermissionsResult`
时间: 2024-09-16 22:04:00 浏览: 55
在Android开发中,当子类覆盖了父类的方法(如Activity的onRequestPermissionsResult()方法),通常需要确保先调用父类的同名方法。这样做是为了保持代码的继承性和防止丢失父类对某些操作的处理。`super.onRequestPermissionsResult()`这一句就是用于调用父类的这个方法,以便能够响应权限请求并传递回调结果给父组件,如果有必要的话,还可以在子类自己的实现上添加额外的逻辑。
这是一个示例代码片段:
```java
@Override
public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
// 在这里添加子类特有的处理
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
// 处理权限被接受的情况
} else {
// 处理权限被拒绝的情况
}
}
```
相关问题
Overriding method should call super.onCreate
As an AI language model, I am not capable of giving personal opinions or beliefs. However, I can provide general information on the topic.
Overriding a method in Android refers to providing a new implementation of a method that already exists in a parent class. When overriding a method, it is generally recommended to call the parent method using the super keyword. This is because the parent implementation may contain important functionality that should not be lost.
For example, when overriding the onCreate method in an Activity, it is important to call super.onCreate to ensure that the Activity is properly initialized.
Here is an example of how to properly override the onCreate method in an Activity:
```
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// Your code here
}
```
By calling super.onCreate, you ensure that the parent implementation of the onCreate method is executed before your own code is executed. This can prevent unexpected behavior and ensure that your Activity is properly initialized.
UnityEditor.ObjectPreview was not disposed properly. Make sure that base.Cleanup is called if overriding the Cleanup method. If you are implementing this in an Editor or EditorWindow, don't forget to call ObjectPreview.Cleanup in OnDisable. UnityEditor.ObjectPreview:Finalize ()
这个错误通常是由于在Unity编辑器中使用`ObjectPreview`类时没有正确处理资源清理导致的。为了解决这个问题,你需要在相应的地方调用`Cleanup`方法。
根据错误信息,你可以尝试以下解决方法:
1. 如果你在自定义的类中重写了`Cleanup`方法,请确保你在该方法中调用了基类的`Cleanup`方法。例如:
```csharp
public class CustomObjectPreview : ObjectPreview
{
protected override void Cleanup()
{
// 清理自定义资源
base.Cleanup(); // 调用基类的Cleanup方法
}
}
```
2. 如果你在`Editor`或`EditorWindow`中使用了`ObjectPreview`,请确保在`OnDisable`方法中调用了`ObjectPreview.Cleanup`。例如:
```csharp
public class CustomEditorWindow : EditorWindow
{
private ObjectPreview objectPreview;
private void OnDisable()
{
if (objectPreview != null)
{
objectPreview.Cleanup();
}
}
}
```
通过在合适的地方调用`Cleanup`方法,你可以正确处理资源清理,避免出现该错误。如果问题仍然存在,你可以进一步检查代码并查看是否有其他未正确处理资源清理的地方。
阅读全文
相关推荐
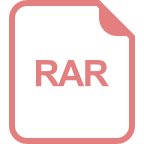
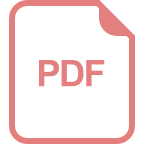
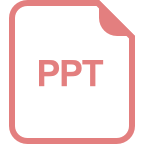









