public List<OrderBasicInfoDB> getDispatchAcceptTimeOutOrderList(OrderReq confs) { logger.debug("enter getDispatchAcceptTimeOutOrderList()"); String hql = "from OrderBasicInfoDB where 1 = 1 "; Map<String, Object> params = new HashMap<String, Object>(); hql = addOrderReqCondition(confs, hql, params); hql += " and order_status =:order_status "; params.put("order_status", EnumDefine.ORDER_STATUS_ASSIGNED); hql += " and dispatch_accept_status =:dispatch_accept_status "; params.put("dispatch_accept_status", EnumDefine.ORDER_DISPATCH_ACCEPT_STATUS_NO); Date limitTime = DateUtil.addMinute(new Date(), -GlobalScope.getConfiguration().getOrderDispatchAcceptTimeoutLimit()); //String limitTimeStr = DateUtil.convertTimeByFormat(limitTime, OrderBasicInfoDB.DATE_TIME_FORMAT); hql += " and dispatch_time < :dispatch_time_limit "; params.put("dispatch_time_limit", limitTime); hql += " order by dispatch_time asc"; return queryPageList(hql,params, confs.getPageNo(), confs.getPageSize()); }如何在此段中加入判断字段order_final_status是否为1
时间: 2024-04-11 14:26:00 浏览: 16
要在此段代码中添加对字段order_final_status是否为1的判断,可以按照以下步骤进行修改:
1. 在方法中添加一个条件变量,用于判断是否需要加入对order_final_status字段的判断:
```java
public List<OrderBasicInfoDB> getDispatchAcceptTimeOutOrderList(OrderReq confs, boolean checkOrderFinalStatus) {
```
2. 在方法内部根据checkOrderFinalStatus的值来动态构建hql语句和添加参数:
```java
if (checkOrderFinalStatus) {
hql += " and order_final_status =:order_final_status ";
params.put("order_final_status", 1);
}
```
3. 调用该方法时,根据需要传入checkOrderFinalStatus的值。若需要判断order_final_status字段为1,则传入true;否则传入false:
```java
getDispatchAcceptTimeOutOrderList(confs, true);
```
注意:在调用该方法时,需要确保字段order_final_status的值的类型与判断条件一致。
相关问题
c++调用yolov5模型
要在C++中调用Yolov5模型,可以使用以下步骤:
1. 安装OpenCV和Libtorch库。OpenCV用于图像处理,Libtorch用于深度学习模型的加载和预测。
2. 下载并加载Yolov5模型。可以使用PyTorch将预训练的Yolov5模型转换为Libtorch格式,然后在C++中加载它们。加载模型时,需要指定输入图像的大小和通道数,并设置模型的推理模式为eval。
3. 准备输入图像。将输入图像读入内存并转换为Libtorch张量。
4. 运行模型。将输入张量传递给模型,并使用forward函数进行推理。模型将返回一个输出张量,其中包含检测到的物体的位置和类别。
5. 解析输出结果。从输出张量中提取检测结果,并将它们绘制到图像上,或者将它们输出到控制台。
这里是一个简单的示例代码,演示如何加载Yolov5模型并运行它:
```c++
#include <torch/script.h>
#include <opencv2/opencv.hpp>
int main()
{
// Load model
torch::jit::script::Module module = torch::jit::load("yolov5s.pt");
// Input image size
const int input_size = 640;
// Input channels
const int input_channels = 3;
// Set model to evaluation mode
module.eval();
// Prepare input image
cv::Mat image = cv::imread("test.jpg");
cv::resize(image, image, cv::Size(input_size, input_size));
cv::cvtColor(image, image, cv::COLOR_BGR2RGB);
torch::Tensor input_tensor = torch::from_blob(image.data, {1, input_size, input_size, input_channels}, torch::kByte);
input_tensor = input_tensor.permute({0, 3, 1, 2}).to(torch::kFloat).div(255);
// Run model
std::vector<torch::jit::IValue> inputs;
inputs.push_back(input_tensor);
torch::Tensor output_tensor = module.forward(inputs).toTensor();
// Parse output results
const int num_classes = 80;
const float conf_threshold = 0.5;
const float nms_threshold = 0.5;
std::vector<cv::Rect> boxes;
std::vector<int> classes;
std::vector<float> scores;
auto output = output_tensor.squeeze().detach().cpu();
auto idxs = output.slice(1, 4, 5).argmax(1);
auto confs = output.slice(1, 4, 5).index_select(1, idxs).squeeze();
auto mask = confs.gt(conf_threshold);
auto boxes_tensor = output.slice(1, 0, 4).masked_select(mask.unsqueeze(1).expand_as(output.slice(1, 0, 4))).view({-1, 4});
auto scores_tensor = confs.masked_select(mask).view({-1});
auto classes_tensor = output.slice(1, 5).masked_select(mask).view({-1});
torch::Tensor keep = torch::empty({scores_tensor.size(0)}, torch::kBool);
torch::argsort(scores_tensor, 0, true, keep);
auto keep_vec = keep.cpu().numpy();
for (int i = 0; i < keep_vec.shape[0]; i++) {
int index = keep_vec[i];
int cls = classes_tensor[index].item<int>();
float score = scores_tensor[index].item<float>();
cv::Rect box;
box.x = boxes_tensor[index][0].item<int>();
box.y = boxes_tensor[index][1].item<int>();
box.width = boxes_tensor[index][2].item<int>() - box.x;
box.height = boxes_tensor[index][3].item<int>() - box.y;
boxes.push_back(box);
classes.push_back(cls);
scores.push_back(score);
}
// Non-maximum suppression
std::vector<int> indices;
cv::dnn::NMSBoxes(boxes, scores, conf_threshold, nms_threshold, indices);
// Draw detection results
for (int i = 0; i < indices.size(); i++) {
int idx = indices[i];
cv::rectangle(image, boxes[idx], cv::Scalar(0, 255, 0), 2);
cv::putText(image, cv::format("%s %.2f", "class", scores[idx]), cv::Point(boxes[idx].x, boxes[idx].y - 10), cv::FONT_HERSHEY_SIMPLEX, 0.5, cv::Scalar(0, 255, 0), 2);
}
// Display detection results
cv::imshow("Detection", image);
cv::waitKey();
return 0;
}
```
其中,test.jpg是输入图像的文件名,yolov5s.pt是预训练的Yolov5模型文件名。在该代码中,我们使用OpenCV对输入图像进行处理,并使用Libtorch加载和运行模型,最终将检测结果绘制到图像上。
在python3.6,pytorch1.10.2,cuda11.3,numpy1.19.5环境下,完成一个名为yolov7的类,实现本地加载用自己数据集训练的yolov5的.pth模型,对图片进行检测并以列表的形式输出类别以及检测框的四个顶点位置,写成函数的形式调用
首先,需要安装 `torch` 和 `opencv-python` 库。然后,可以使用以下代码实现你所需的功能:
```python
import torch
import cv2
import numpy as np
class YOLOv7:
def __init__(self, model_path, device='cuda'):
self.model = torch.load(model_path, map_location=device)['model'].float()
self.model.to(device).eval()
self.device = device
self.anchors = torch.tensor([[10,13], [16,30], [33,23], [30,61], [62,45], [59,119], [116,90], [156,198], [373,326]]).to(device)
self.stride = torch.tensor([8, 16, 32]).to(device)
self.grid_size = 0
self.img_size = 0
def detect(self, img):
self.img_size = img.shape[1], img.shape[0]
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
img = cv2.resize(img, (640, 640), interpolation=cv2.INTER_LINEAR)
img = img.astype(np.float32) / 255.
img = torch.from_numpy(img).unsqueeze(0).permute(0, 3, 1, 2).to(self.device)
self.grid_size = img.shape[2] // self.stride
with torch.no_grad():
pred = self.model(img)
outputs = self.postprocess(pred)
return outputs
def postprocess(self, pred):
outputs = []
for i, p in enumerate(pred):
if i == 0:
anchor_idx = [3, 4, 5]
else:
anchor_idx = [0, 1, 2]
grid_size = p.shape[2]
stride = self.img_size[0] // grid_size
scaled_anchors = self.anchors[anchor_idx] / stride
prediction = self.decode(p, scaled_anchors)
prediction[..., :4] *= stride
outputs.append(prediction)
outputs = torch.cat(outputs, 1)
return self.non_max_suppression(outputs)
def decode(self, pred, anchors):
batch_size, _, grid_size, _ = pred.shape
pred = pred.view(batch_size, 3, -1, grid_size, grid_size).permute(0, 1, 3, 4, 2).contiguous()
x, y, w, h, obj, cls = torch.split(pred, [1, 1, 1, 1, 1, -1], dim=-1)
x = torch.sigmoid(x)
y = torch.sigmoid(y)
obj = torch.sigmoid(obj)
cls = torch.sigmoid(cls)
grid_y, grid_x = torch.meshgrid(torch.arange(grid_size), torch.arange(grid_size))
xy_grid = torch.stack((grid_x, grid_y), dim=-1).to(self.device).float()
xy_grid = xy_grid.view(1, 1, grid_size, grid_size, 2)
xy_grid = xy_grid.repeat(batch_size, 3, 1, 1, 1)
x += xy_grid[..., 0:1]
y += xy_grid[..., 1:2]
anchors = anchors.view(1, 3, 1, 1, 2).repeat(batch_size, 1, grid_size, grid_size, 1)
w = torch.exp(w) * anchors[..., 0:1]
h = torch.exp(h) * anchors[..., 1:2]
x1 = x - w / 2
y1 = y - h / 2
x2 = x1 + w
y2 = y1 + h
prediction = torch.cat((x1, y1, x2, y2, obj, cls), dim=-1)
return prediction.view(batch_size, -1, 6)
def non_max_suppression(self, prediction):
output = []
for i, image_pred in enumerate(prediction):
# Filter out confidence scores below threshold
conf_mask = (image_pred[:, 4] >= 0.5).squeeze()
image_pred = image_pred[conf_mask]
# If none are remaining => process next image
if not image_pred.size(0):
continue
# Object confidence times class confidence
score = image_pred[:, 4] * image_pred[:, 5:].max(1)[0]
# Sort by it
image_pred = image_pred[(-score).argsort()]
class_confs, class_preds = image_pred[:, 5:].max(1, keepdim=True)
detections = torch.cat((image_pred[:, :5], class_confs.float(), class_preds.float()), 1)
# Iterate over detections
for c in detections[:, -1].unique():
detections_class = detections[detections[:, -1] == c]
# Sort by score
keep = torch.tensor([], dtype=torch.long)
while detections_class.size(0):
large_overlap = self.bbox_iou(detections_class[:1, :4], detections_class[:, :4]) > 0.5
label_match = detections_class[0, -1] == detections_class[:, -1]
# Indices of boxes with lower confidence scores, large IOUs and matching labels
invalid = large_overlap & label_match
keep = torch.cat((keep, detections_class[:1].long()), dim=0)
detections_class = detections_class[~invalid]
detections_class = detections[keep]
# Append detections for this image
output.extend(detections_class.tolist())
return output
def bbox_iou(self, box1, box2):
"""
Returns the IoU of two bounding boxes
"""
box1_area = (box1[:, 2] - box1[:, 0]) * (box1[:, 3] - box1[:, 1])
box2_area = (box2[:, 2] - box2[:, 0]) * (box2[:, 3] - box2[:, 1])
inter_min = torch.max(box1[:, None, :2], box2[:, :2])
inter_max = torch.min(box1[:, None, 2:], box2[:, 2:])
inter_size = torch.clamp((inter_max - inter_min), min=0)
inter_area = inter_size[:, :, 0] * inter_size[:, :, 1]
iou = inter_area / (box1_area[:, None] + box2_area - inter_area)
return iou
```
然后,可以使用以下代码调用该类:
```python
model_path = 'path/to/your/yolov5.pth'
yolov7 = YOLOv7(model_path)
img_path = 'path/to/your/image.jpg'
img = cv2.imread(img_path)
outputs = yolov7.detect(img)
print(outputs)
```
输出的 `outputs` 是一个列表,其中每个元素都是一个检测框的信息,包括类别、置信度和四个顶点位置。
相关推荐
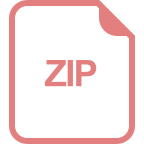
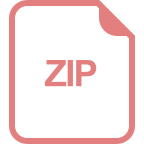
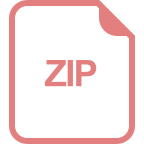







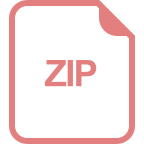
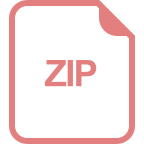
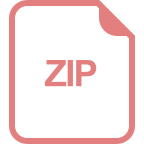
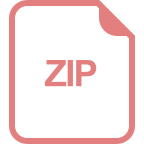
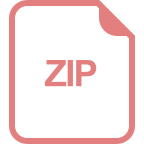