c++解析http post请求 并打印正文数据
时间: 2024-12-16 17:32:01 浏览: 3
在C++中解析HTTP POST请求并打印正文数据通常需要借助第三方库,如`cpprestsdk`(以前称为`WinHttpClient`),它提供了一套完整的HTTP客户端API。以下是使用cpprestsdk的一个简单示例:
```cpp
#include <cpprest/http_client.h>
#include <iostream>
using namespace web;
using namespace web::http;
using namespace concurrency;
void parsePostRequest(const uri &uri) {
http_client client(uri);
// 发送POST请求
auto request_options = http_request::options();
request_options.set_method(methods::post);
pplx::task<http_response> response_task = client.request(request_options);
try {
// 等待响应并处理结果
auto response = await response_task;
if (response.status_code() == status_codes::OK) {
// 获取POST正文
std::string requestBody;
response.read_to_string(requestBody);
// 打印POST正文
std::cout << "POST Request Body:\n" << requestBody << std::endl;
} else {
std::cout << "Error: HTTP status code " << response.status_code() << std::endl;
}
} catch (const std::exception &e) {
std::cerr << "Error: " << e.what() << std::endl;
}
}
int main() {
uri server_uri("http://example.com/api/endpoint"); // 替换为实际服务器地址
parsePostRequest(server_uri);
return 0;
}
```
这个例子首先创建了一个`http_client`实例,然后发送一个POST请求到指定的URL。如果请求成功,响应的正文会被读取并打印出来。
请注意,这只是一个基本的示例,实际应用中你可能还需要处理更复杂的情况,例如错误处理、添加请求头、设置超时等。同时,如果你希望解析JSON或其他特定格式的数据,可能需要额外的库来解析内容。
阅读全文
相关推荐
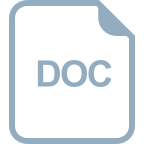
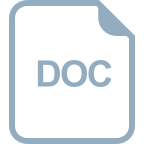
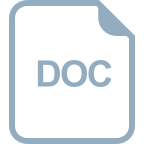
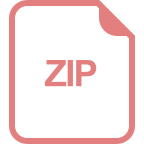
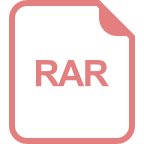
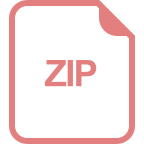
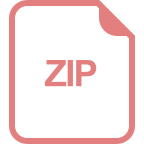
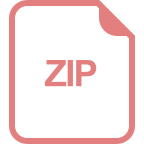
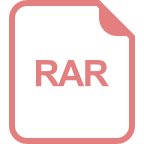
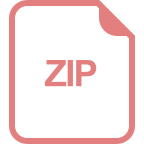
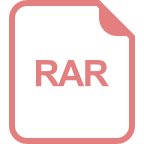
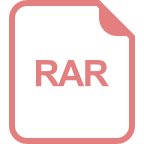
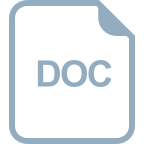





