C语言中设计并实现求解两个向量内积的分治算法
时间: 2024-11-09 20:25:15 浏览: 6
在C语言中,我们可以利用分治算法的思想来设计求解两个向量内积的过程,通常内积也称为点积。分治策略在这里的应用可以分解为递归过程,将复杂的问题分解成更小、相似的部分解决。
首先,让我们明确一下,向量A和B的内积计算公式是:`A[i] * B[i]`,对于n维向量,总和就是 `sum = A[0]*B[0] + A[1]*B[1] + ... + A[n-1]*B[n-1]`。
下面是一个简单的分治方法(实际上这不是分治,因为内积操作不需要分割,它是一个线性操作):
```c
#include <stdio.h>
// 函数声明
double dotProduct(int n, double* vectorA, double* vectorB);
// 主函数
int main() {
int n;
printf("Enter the dimension of vectors: ");
scanf("%d", &n);
double* vectorA = malloc(n * sizeof(double));
double* vectorB = malloc(n * sizeof(double));
// 输入向量元素
printf("Enter elements of vector A: ");
for (int i = 0; i < n; i++) {
scanf("%lf", &vectorA[i]);
}
printf("Enter elements of vector B: ");
for (int i = 0; i < n; i++) {
scanf("%lf", &vectorB[i]);
}
// 计算内积
double result = dotProduct(n, vectorA, vectorB);
printf("The dot product of vectors A and B is: %lf\n", result);
free(vectorA);
free(vectorB);
return 0;
}
// 分治函数(实际这里是个迭代过程而非递归)
double dotProduct(int n, double* vectorA, double* vectorB) {
double sum = 0.0;
for (int i = 0; i < n; i++) {
sum += vectorA[i] * vectorB[i];
}
return sum;
}
```
阅读全文
相关推荐
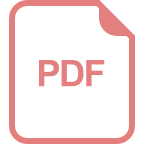
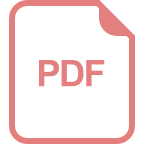
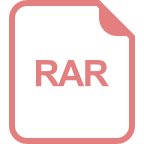
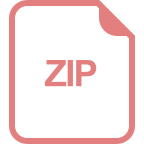
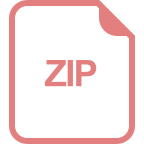
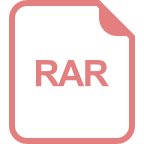
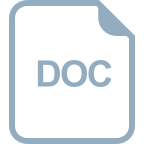
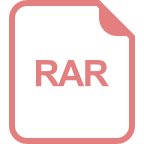
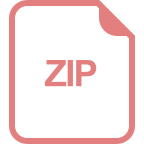
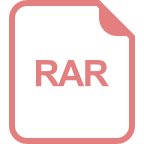
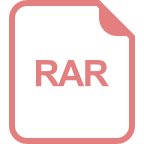
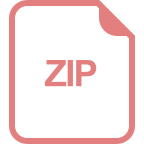
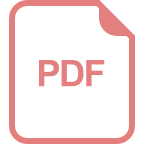
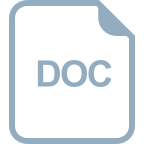
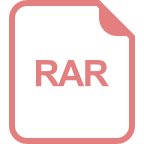
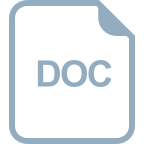
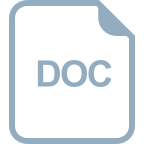