A和B分别是输入的两位BCD加数和被加数;D的低8位是输出的两位BCD数和值;最高位,即D[8]是进位输出。完成实现两位8421BCD码相加的Verilog设计程序。
时间: 2023-12-03 14:43:27 浏览: 63
以下是实现两位8421BCD码相加的Verilog设计程序,包括BCD转换模块、加法器模块和顶层模块:
```
module bcd_to_decimal(
input [3:0] bcd,
output reg [7:0] decimal
);
always @(*) begin
case (bcd)
4'b0000: decimal = 8'd0;
4'b0001: decimal = 8'd1;
4'b0010: decimal = 8'd2;
4'b0011: decimal = 8'd3;
4'b0100: decimal = 8'd4;
4'b0101: decimal = 8'd5;
4'b0110: decimal = 8'd6;
4'b0111: decimal = 8'd7;
4'b1000: decimal = 8'd8;
4'b1001: decimal = 8'd9;
default: decimal = 8'd0;
endcase
end
endmodule
module decimal_to_bcd(
input [7:0] decimal,
output reg [3:0] bcd
);
always @(*) begin
case (decimal)
8'd0: bcd = 4'b0000;
8'd1: bcd = 4'b0001;
8'd2: bcd = 4'b0010;
8'd3: bcd = 4'b0011;
8'd4: bcd = 4'b0100;
8'd5: bcd = 4'b0101;
8'd6: bcd = 4'b0110;
8'd7: bcd = 4'b0111;
8'd8: bcd = 4'b1000;
8'd9: bcd = 4'b1001;
default: bcd = 4'b0000;
endcase
end
endmodule
module bcd_adder(
input [3:0] a,
input [3:0] b,
output reg [3:0] sum,
output reg carry
);
reg [7:0] decimal_a, decimal_b, decimal_sum;
bcd_to_decimal bcd_to_decimal_a(.bcd(a), .decimal(decimal_a));
bcd_to_decimal bcd_to_decimal_b(.bcd(b), .decimal(decimal_b));
always @(*) begin
decimal_sum = decimal_a + decimal_b;
if (decimal_sum > 99) begin
carry = 1;
decimal_sum = decimal_sum - 100;
end else begin
carry = 0;
end
end
decimal_to_bcd decimal_to_bcd_sum(.decimal(decimal_sum), .bcd(sum));
endmodule
module bcd_adder_top(
input [7:0] a,
input [7:0] b,
output reg [8:0] d
);
reg [3:0] a_low, b_low, d_low;
reg [4:0] a_high, b_high, d_high;
reg carry;
bcd_adder bcd_adder_low(.a(a[3:0]), .b(b[3:0]), .sum(d_low), .carry(carry));
bcd_adder bcd_adder_high(.a(a[7:4]), .b(b[7:4]), .sum(d_high), .carry(carry));
always @(*) begin
if (carry) begin
d_high = d_high + 1;
end
end
decimal_to_bcd decimal_to_bcd_d_low(.decimal(d_low), .bcd(d[3:0]));
decimal_to_bcd decimal_to_bcd_d_high(.decimal(d_high), .bcd(d[8:4]));
endmodule
```
顶层模块 `bcd_adder_top` 接收两个8位的BCD数 `a` 和 `b`,将它们拆分成低4位和高4位,分别送入两个 `bcd_adder` 模块中相加。得到的低4位和高4位结果会通过 `decimal_to_bcd` 模块转换成BCD码,并组合成一个9位的BCD数 `d` 输出。在低位相加的过程中,进位会通过 `carry` 变量传递到高位相加中,如果高位相加有进位,则在最高位输出 `1`,否则输出 `0`。
阅读全文
相关推荐
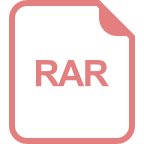
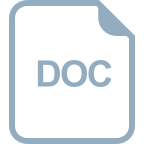

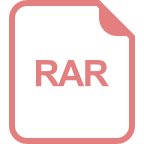











