vue手写实现移动端el-table组件
时间: 2023-10-06 22:06:44 浏览: 187
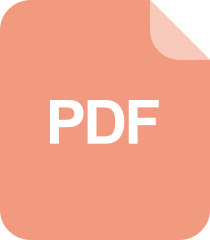
vue项目中将element-ui table表格写成组件的实现代码
好的,我会给您提供一些实现思路和代码示例。
首先,我们可以在组件中定义表头和表格数据的prop,以及一些表格的基本配置参数。如下所示:
```javascript
props: {
columns: {
type: Array,
default: () => []
},
data: {
type: Array,
default: () => []
},
rowKey: {
type: String,
default: 'id'
},
height: {
type: [String, Number],
default: 'auto'
},
pageSize: {
type: Number,
default: 10
},
showPagination: {
type: Boolean,
default: true
},
showIndex: {
type: Boolean,
default: true
},
showHeader: {
type: Boolean,
default: true
},
border: {
type: Boolean,
default: true
}
},
```
接下来,我们可以利用计算属性来实现表格的响应式布局。例如,计算列宽度的方法可以如下所示:
```javascript
computed: {
tableWidth() {
return this.columns.reduce((total, column) => {
return total + (column.width || 100)
}, 0)
},
tableHeight() {
if (this.height === 'auto') {
return 'auto'
} else {
return `${this.height}px`
}
},
cellStyle() {
return {
width: `${(100 / this.columns.length).toFixed(2)}%`
}
},
thStyle() {
return {
width: `${(100 / this.columns.length).toFixed(2)}%`
}
}
},
```
然后,我们可以在模板中渲染表格的各个部分,如表格头部、表格内容、分页等。例如,表格头部的渲染代码可以如下所示:
```html
<template>
<table class="el-table" :style="{ width: tableWidth, height: tableHeight, border: border ? '1px solid #ccc' : 'none' }">
<colgroup>
<col v-if="showIndex" style="width: 50px;">
<col v-for="(column, index) in columns" :key="index" :style="cellStyle">
</colgroup>
<thead v-show="showHeader">
<tr>
<th v-if="showIndex">序号</th>
<th v-for="(column, index) in columns" :key="index" :style="thStyle">{{ column.title }}</th>
</tr>
</thead>
<tbody>
...
</tbody>
</table>
</template>
```
最后,我们可以在组件中实现表格的分页、排序、搜索等功能,以及一些交互效果。例如,分页功能的实现代码可以如下所示:
```javascript
computed: {
pageCount() {
return Math.ceil(this.data.length / this.pageSize)
},
currentData() {
const start = (this.currentPage - 1) * this.pageSize
const end = start + this.pageSize
return this.data.slice(start, end)
}
},
methods: {
handlePageChange(currentPage) {
this.currentPage = currentPage
}
}
```
```html
<template>
<div>
<table class="el-table" :style="{ width: tableWidth, height: tableHeight, border: border ? '1px solid #ccc' : 'none' }">
...
</table>
<el-pagination v-if="showPagination"
:current-page.sync="currentPage"
:page-size="pageSize"
:total="data.length"
@current-change="handlePageChange">
</el-pagination>
</div>
</template>
```
以上就是一个简单的移动端el-table组件的实现思路和代码示例。希望能对您有所帮助。
阅读全文