用python实现抽取excel中的数据
时间: 2023-05-20 21:04:31 浏览: 246
可以使用Python中的pandas库来实现抽取excel中的数据。具体步骤如下:
1. 安装pandas库:在命令行中输入pip install pandas进行安装。
2. 导入pandas库:在Python代码中使用import pandas语句导入pandas库。
3. 使用pandas读取excel文件:使用pandas的read_excel函数读取excel文件,例如:df = pandas.read_excel('example.xlsx')。
4. 抽取数据:使用pandas的数据操作函数,例如df['列名']可以抽取某一列的数据,df.loc[行索引, 列索引]可以抽取某一行或某一单元格的数据。
5. 处理数据:对抽取的数据进行处理,例如进行计算、筛选等操作。
6. 输出数据:将处理后的数据输出到文件或者打印到控制台,例如使用pandas的to_excel函数将数据输出到excel文件中。
以上就是用Python实现抽取excel中的数据的基本步骤。
相关问题
python抽取excel指定数据
要抽取Excel中的指定数据,可以使用以下方法:
首先,需要安装Excel读取数据的库xlrd。然后,获取Excel文件的位置并读取进来。接下来,读取指定的行和列的内容,并将其存储在列表中。
以下是完整的Python程序代码:
```python
import xlrd
from xlrd import xldate_as_tuple
import datetime
# 导入需要读取的Excel表格的路径
data1 = xlrd.open_workbook(r'C:\Users\NHT\Desktop\Data\\test.xlsx')
table = data1.sheets()[0]
# 创建一个空列表,存储Excel的数据
tables = []
# 将Excel表格内容导入到tables列表中
def import_excel(excel):
for rown in range(excel.nrows):
array = {'road_name':'','bus_plate':'','timeline':'','road_type':'','site':''}
array['road_name'] = table.cell_value(rown,0)
array['bus_plate'] = table.cell_value(rown,1)
if table.cell(rown,2).ctype == 3:
date = xldate_as_tuple(table.cell(rown,2).value,0)
array['timeline'] = datetime.datetime(*date)
array['road_type'] = table.cell_value(rown,3)
array['site'] = table.cell_value(rown,4)
tables.append(array)
if __name__ == '__main__':
# 将Excel表格的内容导入到列表中
import_excel(table)
# 验证Excel文件存储到列表中的数据
for i in tables:
print(i)
```
在上述代码中,我们首先导入需要读取的Excel表格的路径,然后创建一个空列表`tables`来存储Excel的数据。接下来,我们定义了一个函数`import_excel`,用于将Excel表格内容导入到`tables`列表中。最后,在`if __name__ == '__main__'`的代码块中,我们调用`import_excel`函数将Excel表格的内容导入到列表中,并打印出验证结果。
注意,你需要将代码中的Excel文件路径修改为你实际的文件路径。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [python如何读取excel表数据](https://blog.csdn.net/ekcchina/article/details/124446961)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
利用python按照时间跨度抽取excel表格中的数据代码
抽取 Excel 表格中的数据可以使用 Python 中的 pandas 库。以下是一个示例代码,可以通过指定起始时间和结束时间来按照时间跨度抽取表格数据:
```python
import pandas as pd
# 读取 Excel 表格
df = pd.read_excel('your_file.xlsx')
# 将时间列转换为 datetime 类型
df['time'] = pd.to_datetime(df['time'])
# 按照时间跨度抽取数据
start_time = '2022-09-01'
end_time = '2022-09-30'
mask = (df['time'] >= start_time) & (df['time'] <= end_time)
filtered_df = df.loc[mask]
# 将抽取的数据保存为新的 Excel 表格
filtered_df.to_excel('filtered_file.xlsx', index=False)
```
在这个示例代码中,我们首先使用 `pd.read_excel()` 函数读取 Excel 表格数据,并将时间列转换为 datetime 类型。然后,我们指定起始时间和结束时间,并使用布尔掩码从原始数据中抽取符合条件的数据。最后,我们将抽取的数据保存为新的 Excel 表格,以备后续使用。
阅读全文
相关推荐
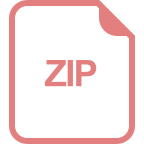
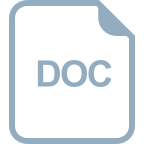
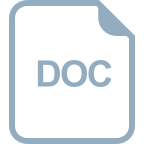
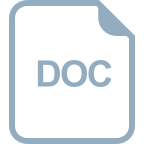



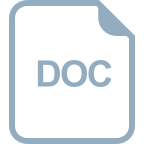



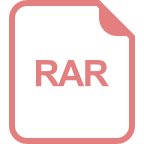
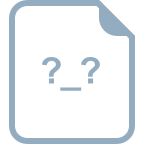
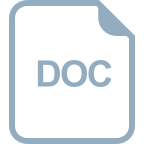
