用java设计图形用户界面,功能要求: 1. 要求在文本框中输入有关信息,单击“添加”按钮,在下面的多行文本域中显示输入的的信 息(如姓名、性别、年龄、爱好); 2. 单击“读取”按钮将文本文件中的信息显示到多行文本域中。 3. 单击“保存”按钮将把多行文本域中的内容保存到文本文件中,并退出窗口。
时间: 2024-03-23 08:36:44 浏览: 58
好的,你的需求可以通过使用Java Swing来设计一个图形用户界面程序来实现。下面是一个示例代码:
```java
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
public class MyGUI extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JTextField nameField, genderField, ageField, hobbyField;
private JTextArea infoArea;
private JButton addButton, readButton, saveButton;
public MyGUI() {
super("My GUI");
// create the GUI components
nameField = new JTextField(20);
genderField = new JTextField(20);
ageField = new JTextField(20);
hobbyField = new JTextField(20);
infoArea = new JTextArea(10, 30);
addButton = new JButton("Add");
readButton = new JButton("Read");
saveButton = new JButton("Save");
// add the components to the content pane
JPanel inputPanel = new JPanel();
inputPanel.add(nameField);
inputPanel.add(genderField);
inputPanel.add(ageField);
inputPanel.add(hobbyField);
inputPanel.add(addButton);
JPanel buttonPanel = new JPanel();
buttonPanel.add(readButton);
buttonPanel.add(saveButton);
getContentPane().add(inputPanel, BorderLayout.NORTH);
getContentPane().add(new JScrollPane(infoArea), BorderLayout.CENTER);
getContentPane().add(buttonPanel, BorderLayout.SOUTH);
// add action listeners to the buttons
addButton.addActionListener(this);
readButton.addActionListener(this);
saveButton.addActionListener(this);
// set the frame properties
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 300);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
// get the input values
String name = nameField.getText();
String gender = genderField.getText();
String age = ageField.getText();
String hobby = hobbyField.getText();
// add the values to the info area
infoArea.append(name + "\t" + gender + "\t" + age + "\t" + hobby + "\n");
// clear the input fields
nameField.setText("");
genderField.setText("");
ageField.setText("");
hobbyField.setText("");
} else if (e.getSource() == readButton) {
// read the file and display the contents in the info area
try {
BufferedReader br = new BufferedReader(new FileReader("info.txt"));
String line;
while ((line = br.readLine()) != null) {
infoArea.append(line + "\n");
}
br.close();
} catch (IOException ex) {
ex.printStackTrace();
}
} else if (e.getSource() == saveButton) {
// save the contents of the info area to the file
try {
BufferedWriter bw = new BufferedWriter(new FileWriter("info.txt"));
bw.write(infoArea.getText());
bw.close();
} catch (IOException ex) {
ex.printStackTrace();
}
// exit the program
System.exit(0);
}
}
public static void main(String[] args) {
new MyGUI();
}
}
```
这个程序创建了一个包含文本框、多行文本域和按钮的图形用户界面。用户可以在文本框中输入信息,然后单击“添加”按钮将信息添加到多行文本域中。用户还可以单击“读取”按钮将文件中的信息显示在多行文本域中,或者单击“保存”按钮将多行文本域中的内容保存到文件中并退出程序。
阅读全文
相关推荐
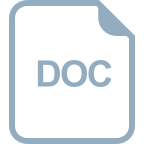
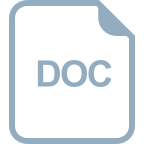
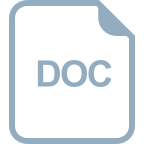


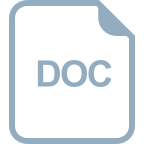
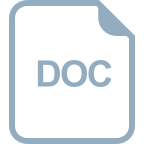
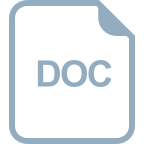
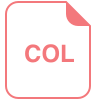
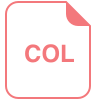

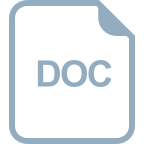
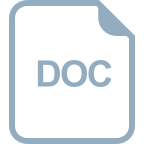
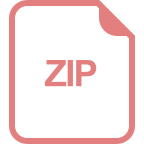
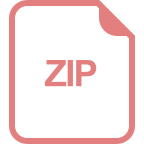
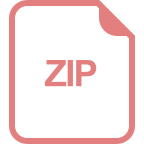
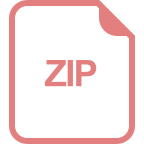
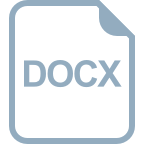
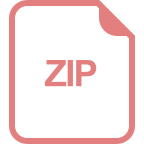