代码生成(java的快速排序怎么写
时间: 2023-08-31 10:36:16 浏览: 123
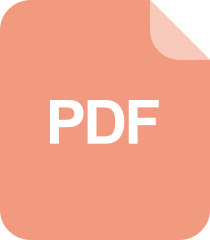
比较排序之快速排序(实例代码)
### 回答1:
下面是java语言实现的快速排序算法:
public static void quickSort(int[] arr, int left, int right) {
if(left < right) {
int partitionIndex = partition(arr, left, right);
quickSort(arr, left, partitionIndex-1);
quickSort(arr, partitionIndex+1, right);
}
}
private static int partition(int[] arr, int left, int right) {
int pivot = left;
int index = pivot + 1;
for(int i = index; i <= right; i++) {
if(arr[i] < arr[pivot]) {
swap(arr, i, index);
index++;
}
}
swap(arr, pivot, index - 1);
return index-1;
}
private static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
### 回答2:
快速排序是一种常用的排序算法,其基本思想是通过选择一个基准元素,将待排序数组分为两个子数组,其中一个子数组中的元素都小于基准元素,另一个子数组中的元素都大于基准元素,然后对这两个子数组进行递归的快速排序。
下面是Java中实现快速排序的代码:
```java
public class QuickSort {
public static void quickSort(int[] array, int low, int high) {
if (low < high) {
int pivotIndex = partition(array, low, high);
quickSort(array, low, pivotIndex - 1);
quickSort(array, pivotIndex + 1, high);
}
}
private static int partition(int[] array, int low, int high) {
int pivot = array[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (array[j] < pivot) {
i++;
swap(array, i, j);
}
}
swap(array, i + 1, high);
return i + 1;
}
private static void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
public static void main(String[] args) {
int[] array = {6, 3, 8, 2, 9, 1};
quickSort(array, 0, array.length - 1);
for (int num : array) {
System.out.print(num + " ");
}
}
}
```
这段代码中,`quickSort`方法是用于调用快速排序的入口,它接收一个待排序的数组以及数组的起始位置和结束位置作为参数。`quickSort`方法中先选择一个基准元素并通过`partition`方法对数组进行划分,然后对划分后的两个子数组分别再次调用`quickSort`方法进行递归排序。
`partition`方法中定义了一个指针`i`,用于记录小于基准元素的子数组的右边界。遍历待排序数组时,如果当前元素小于基准元素,则将`i`向右移动一位,并将当前元素与`i`交换,以保证小于基准元素的子数组的右边界一直处于`i`的右侧。最后,将基准元素与`i+1`位置的元素交换,使得基准元素位于正确的位置上,并返回基准元素的索引。
最后,在`main`方法中,我们定义一个待排序数组并调用`quickSort`方法对其进行排序,并打印排序后的结果。
这就是一种常见的实现快速排序的方法。快速排序的时间复杂度为O(nlogn),是一种高效的排序算法。
### 回答3:
快速排序是一种高效的排序算法,在Java中可以通过递归来实现。下面是一个简单的快速排序的实现示例:
```java
public class QuickSort {
public void quickSort(int[] arr, int low, int high) {
if (low < high) {
// 选择一个基准元素
int pivotIndex = partition(arr, low, high);
// 对基准元素左边的子数组进行快排
quickSort(arr, low, pivotIndex - 1);
// 对基准元素右边的子数组进行快排
quickSort(arr, pivotIndex + 1, high);
}
}
private int partition(int[] arr, int low, int high) {
// 选择最右边的元素作为基准元素
int pivot = arr[high];
int i = low - 1; // 记录小于基准的元素位置
for (int j = low; j < high; j++) {
// 如果当前元素比基准元素小,则将其交换到i的位置
if (arr[j] < pivot) {
i++;
swap(arr, i, j);
}
}
// 将基准元素交换到正确的位置
swap(arr, i + 1, high);
return i + 1;
}
private void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
public static void main(String[] args) {
int[] arr = {5, 2, 8, 9, 1, 3};
QuickSort quickSort = new QuickSort();
quickSort.quickSort(arr, 0, arr.length - 1);
for (int num : arr) {
System.out.print(num + " ");
}
}
}
```
代码中的`quickSort`方法接受一个整型数组、数组的起始索引low和终止索引high作为参数,用于对数组进行快速排序。`partition`方法用于进行划分,选择最右边的元素作为基准元素,将小于基准的元素放在左边,大于基准的元素放在右边,并返回基准元素的位置。`swap`方法用于交换两个元素的值。
在`main`方法中,首先创建一个包含一些无序元素的数组,然后创建`QuickSort`对象,调用`quickSort`方法进行排序。最后打印排序后的结果。
阅读全文
相关推荐
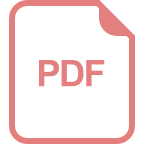
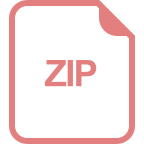







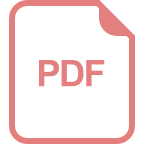
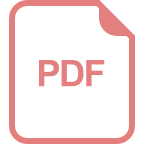
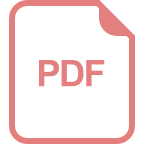
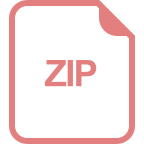
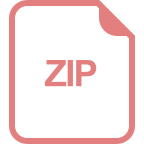
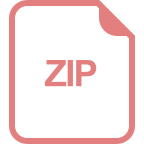
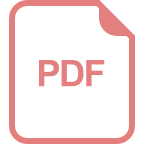