梯度下降法线性回归预测房价源代码
时间: 2023-10-08 19:08:02 浏览: 39
以下是使用梯度下降法实现线性回归预测房价的 Python 代码:
```python
import numpy as np
def gradient_descent(X, y, alpha=0.01, num_iters=1000):
"""
梯度下降法实现线性回归
参数:
X -- 输入特征矩阵,大小为 (m, n),其中 m 为样本数,n 为特征数
y -- 输出结果,大小为 (m, 1)
alpha -- 学习率
num_iters -- 迭代次数
返回:
theta -- 训练得到的参数,大小为 (n, 1)
J_history -- 每次迭代计算得到的代价函数值
"""
m, n = X.shape
theta = np.zeros((n, 1))
J_history = np.zeros((num_iters, 1))
for i in range(num_iters):
# 计算预测值
h = np.dot(X, theta)
# 计算误差
error = h - y
# 计算梯度
grad = np.dot(X.T, error) / m
# 更新参数
theta = theta - alpha * grad
# 计算代价函数
J_history[i] = np.sum(np.power(error, 2)) / (2 * m)
return theta, J_history
# 加载数据
data = np.loadtxt('housing_data.txt', delimiter=',')
X = data[:, :-1]
y = data[:, -1:]
m, n = X.shape
# 特征缩放
mu = np.mean(X, axis=0)
sigma = np.std(X, axis=0)
X = (X - mu) / sigma
# 添加一列常数项
X = np.hstack((np.ones((m, 1)), X))
# 初始化参数
theta = np.zeros((n + 1, 1))
# 训练模型
theta, J_history = gradient_descent(X, y, alpha=0.01, num_iters=1000)
# 预测房价
X_test = np.array([1650, 3])
X_test = (X_test - mu) / sigma
X_test = np.hstack((1, X_test))
price = np.dot(X_test, theta)
print('预测房价:', price[0])
```
其中,housing_data.txt 是包含房屋面积和卧室数目等特征以及房价的数据集,每行数据格式为:面积(单位:平方英尺),卧室数目,房价(单位:美元)。
相关推荐
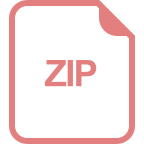














