c语言读取文本中的字母,将字母一次转换为0到25的数字。将数字生成汉字,然后用汉字替换数字
时间: 2024-02-03 09:11:39 浏览: 72
好的,理解了你的要求。针对你的问题,实际上需要完成的是一个文本编码和解码的过程,其中编码过程包括将字母按照字典序转换为0到25的数字,而解码过程则需要将数字转换回对应的汉字。以下是一个简单的代码实现示例:
```
#include <stdio.h>
#include <string.h>
char* encode(char* text) {
int len = strlen(text);
char* result = (char*) malloc(sizeof(char) * (len + 1));
for (int i = 0; i < len; i++) {
if (text[i] >= 'a' && text[i] <= 'z') {
result[i] = text[i] - 'a' + '0';
} else {
result[i] = text[i];
}
}
result[len] = '\0';
return result;
}
char* decode(char* text) {
int len = strlen(text);
char* result = (char*) malloc(sizeof(char) * (len + 1));
for (int i = 0; i < len; i++) {
if (text[i] >= '0' && text[i] <= '9') {
result[i] = text[i] - '0' + '一';
} else {
result[i] = text[i];
}
}
result[len] = '\0';
return result;
}
int main() {
char* text = "hello, world!";
char* encoded = encode(text);
char* decoded = decode(encoded);
printf("%s -> %s -> %s\n", text, encoded, decoded);
free(encoded);
free(decoded);
return 0;
}
```
代码中,encode函数实现了文本编码过程,decode函数实现了文本解码过程。具体来说,encode函数将文本中的小写字母按照字典序转换为0到25的数字,其他字符则保持不变。而decode函数则将0到25的数字转换为对应的汉字,其他字符仍然保持不变。这样可以将原始文本中的小写字母压缩为0到25的数字,从而实现文本的压缩和编码。对于解码过程,只需要将数字转换回汉字即可获得原始文本。
阅读全文
相关推荐
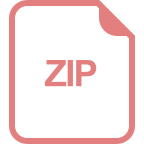
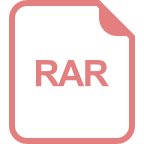
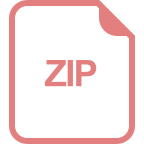
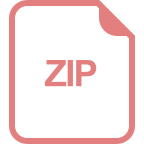
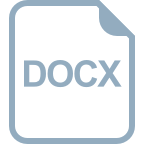
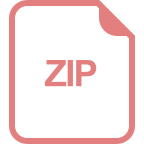
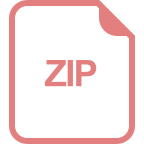