定义类point求两点距离
时间: 2023-05-19 22:04:39 浏览: 103
好的,这是一个简单的类point的定义,可以用来计算两点之间的距离:
```python
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def distance(self, other):
dx = self.x - other.x
dy = self.y - other.y
return (dx ** 2 + dy ** 2) ** 0.5
```
使用方法:
```python
p1 = Point(0, 0)
p2 = Point(3, 4)
print(p1.distance(p2)) # 输出 5.0
```
希望这个回答能够帮到你!
相关问题
用C++定义一个类point,求两点之间的距离
好的,以下是C语言定义一个类point并求两点之间距离的示例代码:
```
#include <stdio.h>
#include <math.h>
struct Point {
int x;
int y;
};
typedef struct Point Point;
double distance(Point p1, Point p2) {
int dx = p2.x - p1.x;
int dy = p2.y - p1.y;
return sqrt(dx*dx + dy*dy);
}
int main() {
Point p1 = {1, 2};
Point p2 = {4, 6};
double dist = distance(p1, p2);
printf("The distance between p1 and p2 is %lf\n", dist);
return 0;
}
```
在这个示例代码中,我们首先定义了一个名为Point的结构体,包含了两个整型成员x和y,代表了一个点的坐标。然后我们使用typedef定义了一个别名Point,方便使用。
接着,我们定义了一个名为distance的函数,该函数接收两个Point类型的参数,计算并返回它们之间的距离。这里我们使用勾股定理计算距离,即sqrt(dx*dx + dy*dy)。
最后,在main函数中我们定义了两个Point类型的对象p1和p2,分别初始化为(1,2)和(4,6),然后调用distance函数计算它们的距离,并打印输出结果。
希望对您有帮助,如有问题请随时提出。
设置点类point求两点之间距离,利用成员函数
可以使用以下代码实现:
```cpp
#include <iostream>
#include <cmath>
class Point {
public:
Point(double x, double y) : x_(x), y_(y) {}
double distanceTo(const Point& other) const {
double dx = x_ - other.x_;
double dy = y_ - other.y_;
return std::sqrt(dx * dx + dy * dy);
}
private:
double x_;
double y_;
};
int main() {
Point p1(0, 0);
Point p2(3, 4);
std::cout << "Distance between p1 and p2 is " << p1.distanceTo(p2) << std::endl;
return 0;
}
```
这个程序定义了一个点类 Point,其中包含了两个成员变量 x 和 y,表示点的坐标。类中还定义了一个成员函数 distanceTo,用于计算当前点与另一个点之间的距离。在主函数中,我们创建了两个点 p1 和 p2,并调用 p1 的 distanceTo 函数来计算两点之间的距离。
相关推荐
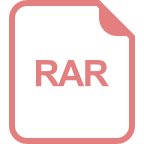
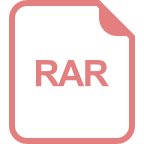
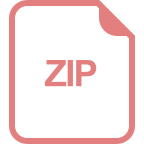












