用C语言代码实现圆弧插补并加上注释
时间: 2023-08-31 20:42:03 浏览: 478
### 回答1:
可以参考下面的代码:
/* 圆弧插补算法 */
#include <stdio.h>
#include <math.h>
// 参数说明:
// xi,yi: 起始点坐标
// xf,yf: 终点坐标
// R: 圆弧半径
// dir: 圆弧方向,1顺时针,-1逆时针
float arc_interp(float xi, float yi, float xf, float yf, float R, int dir)
{
// 中间点坐标
float xm, ym;
// 弧长
float L;
// 角度变量
float theta;
// 判断方向,逆时针还是顺时针
int sign;
// 计算中间点坐标
xm = (xi + xf) / 2;
ym = (yi + yf) / 2;
// 计算弧长
L = sqrt((xi - xf) * (xi - xf) + (yi - yf) * (yi - yf));
// 计算弧度
theta = 2 * asin(L / (2 * R));
// 判断插补方向
sign = (xi - xm) * (yf - ym) - (xf - xm) * (yi - ym);
if (sign > 0)
{
if (dir > 0)
sign = 1;
else
sign = -1;
}
else
{
if (dir > 0)
sign = -1;
else
sign = 1;
}
// 返回角度
return sign * theta;
}
### 回答2:
下面是一个使用C语言代码实现圆弧插补的示例,并带有相应的注释:
```c
#include <stdio.h>
#include <math.h>
// 定义圆弧插补函数
void arcInterpolation(float xCenter, float yCenter, float radius, float startAngle, float endAngle, float stepSize)
{
float currentAngle = startAngle; // 当前角度初始化为起始角度
// 循环遍历从起始角度到终止角度的每一个步长
while (currentAngle <= endAngle)
{
// 计算当前步长位置的x和y坐标
float x = xCenter + radius * cos(currentAngle);
float y = yCenter + radius * sin(currentAngle);
// 在这里可以做一些具体控制命令,如输出位置数据到机器人控制器等
// 更新当前角度
currentAngle += stepSize;
}
}
int main()
{
float xCenter = 2.0; // 圆心x坐标
float yCenter = 2.0; // 圆心y坐标
float radius = 1.0; // 圆弧半径
float startAngle = 0.0; // 圆弧起始角度
float endAngle = M_PI; // 圆弧终止角度,这里使用了数学库中的PI常量
float stepSize = 0.1; // 步长大小,决定圆弧插补的精度
// 调用圆弧插补函数
arcInterpolation(xCenter, yCenter, radius, startAngle, endAngle, stepSize);
return 0;
}
```
此代码实现了圆弧插补的功能,通过计算每个步长位置的x和y坐标,可以在机器人控制器或其他相关设备上使用这些位置数据进行控制。通过调整起始角度、终止角度和步长大小,可以控制圆弧插补的精度。请根据自己的具体需求修改代码中的参数。
### 回答3:
下面是一个使用C语言代码实现圆弧插补的示例,并附有注释:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265
typedef struct {
float x; // 圆心x坐标
float y; // 圆心y坐标
} Point;
// 计算两点之间的距离
float distance(Point p1, Point p2) {
return sqrt(pow((p2.x - p1.x), 2) + pow((p2.y - p1.y), 2));
}
// 计算角度的弧度值
float toRadian(float degree) {
return degree * (PI / 180.0);
}
// 根据圆心、半径、起始角和终止角计算圆弧上的点的坐标
Point getArcPoint(Point center, float radius, float startAngle, float endAngle) {
Point arcPoint;
// 将角度转换为弧度
float radian1 = toRadian(startAngle);
float radian2 = toRadian(endAngle);
// 计算圆弧上的点的x坐标和y坐标
arcPoint.x = center.x + radius * cos(radian2);
arcPoint.y = center.y + radius * sin(radian2);
return arcPoint;
}
int main() {
Point center = { 0, 0 }; // 圆心坐标
float radius = 5.0; // 半径
float startAngle = 0.0; // 起始角度
float endAngle = 90.0; // 终止角度
float step = 1.0; // 步长
Point currentPoint = getArcPoint(center, radius, startAngle, endAngle); // 当前点的坐标
// 通过循环逐步增加角度,计算圆弧上的点的坐标并输出
while (endAngle - startAngle >= step) {
printf("x: %f, y: %f\n", currentPoint.x, currentPoint.y);
// 更新起始角度和终止角度
startAngle += step;
endAngle -= step;
// 计算当前点的坐标
currentPoint = getArcPoint(center, radius, startAngle, endAngle);
}
return 0;
}
```
以上代码实现了一个简单的圆弧插补,通过给定圆心、半径、起始角度和终止角度,计算并输出圆弧上的点的坐标。步长step表示逐步增加的角度值。代码中的注释解释了每一步的操作。
阅读全文
相关推荐
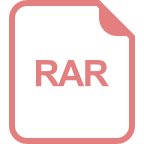
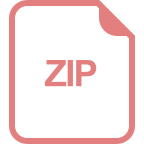
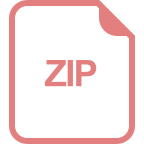















