优化代码def batch_analysis(base_info): """ 算法模块调用函数 :param base_info: :return: """ # set uni-result output headers with open('../../utils/outputs.yaml', 'r') as f: out_headers = yaml.load(f, Loader=yaml.Loader)['algo_module_output'] result_to_classification = {} sns.set_theme(style="white", palette=None) switches = base_info['switches'] solarPV = LfpData(base_info['FileName'], base_info['paths'][0], base_info['paths'][1], base_info['BattMaker'], base_info['BattType']) vin, equipment_result_path, report_path, abnormal_path, stat_path = solarPV.initialization() temp, SOC, OCV, paths = solarPV.get_data(equipment_result_path, base_info['date_assign'][0], base_info['date_assign'][1]) cluster_name = temp['cluster'] print(f"\033[0;31;42m cluster {cluster_name} data imported. \033[0m") # 重点信息【数据已经导入完成】:红色字体绿色背景 # analysis on module data for m_esn in tqdm(temp['modules'].keys()): data_module = {'mod_esn': m_esn, 'data': temp['modules'][m_esn], 'n_volt_probe': temp['n_volt_probe'], 'n_temp_probe': temp['n_temp_probe']} print(f"module_id: {m_esn}, total rows: {len(data_module['data'])}") print(f'\033[0;31;42m module {m_esn} start process... \033[0m') # module全生命周期可视化 lifecycle_visual(m_esn, data_module, paths, switches) # 一致性算法模块 ica_analysis(m_esn, data_module, paths, base_info, out_headers) # 阈值告警算法 threshold_warning(m_esn, data_module, paths, base_info, out_headers) # 采样异常检测算法 # 内/外短路算法 # 故障分类分级算法 del data_module print(f"\033[0;31;42m Module {m_esn}: Cloud BMS Analysis completed. \033[0m") del temp return
时间: 2024-01-10 16:04:56 浏览: 26
可以考虑对代码进行如下优化:
1. 将函数内的注释改为函数外的文档字符串,方便查看函数说明。
2. 将一些常量、字符串放在函数外面作为全局变量,避免反复读取文件或字符串生成对象。
3. 使用 `if __name__ == '__main__'` 来保证模块被导入时不会执行代码。
4. 对于循环中的 `del` 操作,可以考虑使用 `with` 语句来自动关闭资源。
5. 可以将循环中的函数调用改为函数列表,使用循环来遍历调用,使代码更加简洁易读。
6. 建议使用 logging 模块来替代 print 函数,以便更好地管理日志。
修改后的代码如下:
```
import yaml
import logging
import sns
from tqdm import tqdm
from lifecycle_visual import lifecycle_visual
from ica_analysis import ica_analysis
from threshold_warning import threshold_warning
from lfp_data import LfpData
# 常量、字符串等全局变量
OUT_HEADERS = None
def load_out_headers():
with open('../../utils/outputs.yaml', 'r') as f:
return yaml.load(f, Loader=yaml.Loader)['algo_module_output']
def batch_analysis(base_info):
"""
算法模块调用函数
:param base_info:
:return:
"""
global OUT_HEADERS
if OUT_HEADERS is None:
OUT_HEADERS = load_out_headers()
sns.set_theme(style="white", palette=None)
switches = base_info['switches']
solarPV = LfpData(base_info['FileName'], base_info['paths'][0], base_info['paths'][1],
base_info['BattMaker'], base_info['BattType'])
vin, equipment_result_path, report_path, abnormal_path, stat_path = solarPV.initialization()
temp, SOC, OCV, paths = solarPV.get_data(equipment_result_path, base_info['date_assign'][0],
base_info['date_assign'][1])
cluster_name = temp['cluster']
logging.info(f"cluster {cluster_name} data imported.")
# analysis on module data
for m_esn in tqdm(temp['modules'].keys()):
data_module = {'mod_esn': m_esn, 'data': temp['modules'][m_esn],
'n_volt_probe': temp['n_volt_probe'], 'n_temp_probe': temp['n_temp_probe']}
logging.info(f"module_id: {m_esn}, total rows: {len(data_module['data'])}")
logging.info(f'module {m_esn} start process...')
# module全生命周期可视化
lifecycle_visual(m_esn, data_module, paths, switches)
# 一致性算法模块
ica_analysis(m_esn, data_module, paths, base_info, OUT_HEADERS)
# 阈值告警算法
threshold_warning(m_esn, data_module, paths, base_info, OUT_HEADERS)
# 采样异常检测算法
# 内/外短路算法
# 故障分类分级算法
logging.info(f"Module {m_esn}: Cloud BMS Analysis completed.")
# 删除临时数据,使用 with 语句自动关闭资源
with data_module['data'] as f:
del f
with temp as f:
del f
return
if __name__ == '__main__':
batch_analysis(base_info)
```
相关推荐
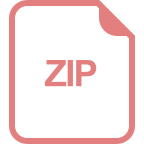















