用springboot框架写一个评论功能,需要具体的代码
时间: 2023-12-11 12:05:56 浏览: 69
好的,下面是一个简单的评论功能的示例代码,使用了Spring Boot框架和MySQL数据库。
首先,我们需要创建一个Comment实体类,用于表示评论的信息:
```
@Entity
@Table(name = "comments")
public class Comment {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String content;
@Column(nullable = false)
private LocalDateTime createdAt;
// 省略getter和setter方法
}
```
接下来,我们需要创建一个CommentRepository接口,用于操作MySQL数据库中的comments表:
```
public interface CommentRepository extends JpaRepository<Comment, Long> {
}
```
然后,我们需要创建一个CommentService类,用于实现评论功能的具体逻辑:
```
@Service
public class CommentService {
@Autowired
private CommentRepository commentRepository;
public void addComment(String content) {
Comment comment = new Comment();
comment.setContent(content);
comment.setCreatedAt(LocalDateTime.now());
commentRepository.save(comment);
}
public List<Comment> getAllComments() {
return commentRepository.findAll();
}
}
```
最后,我们需要创建一个CommentController类,用于接收前端请求并调用CommentService中的方法:
```
@RestController
@RequestMapping("/comments")
public class CommentController {
@Autowired
private CommentService commentService;
@PostMapping
public void addComment(@RequestBody Map<String, String> request) {
String content = request.get("content");
commentService.addComment(content);
}
@GetMapping
public List<Comment> getAllComments() {
return commentService.getAllComments();
}
}
```
现在,我们已经完成了一个简单的评论功能,可以使用Postman等工具测试接口的功能。当然,这只是一个示例代码,实际的项目中可能需要更加完善的功能和处理逻辑。
阅读全文
相关推荐
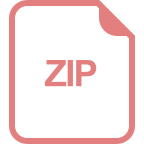
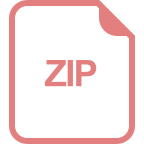
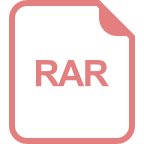
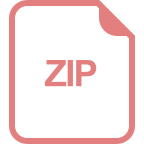
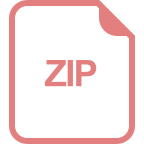
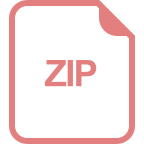
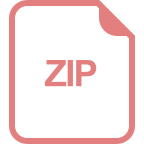
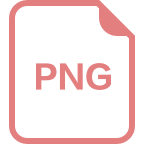
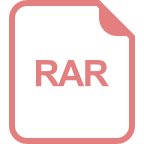
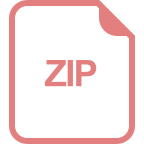
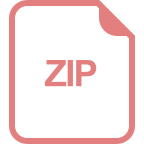
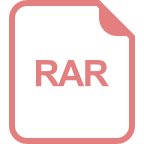
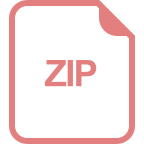
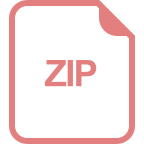
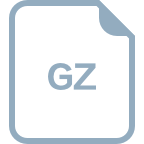
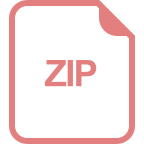
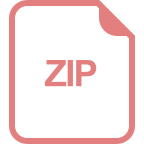