用c语言编写SHA-1算法
时间: 2024-02-11 14:07:15 浏览: 97
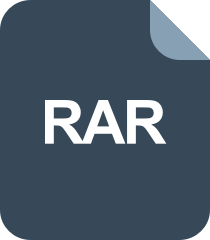
用C语言实现SHA-1算法

以下是一个用C语言编写的SHA-1算法的示例代码:
```c
#include <stdio.h>
#include <stdint.h>
#include <string.h>
// 定义常量
#define SHA1_BLOCK_SIZE 20
// 定义函数
void sha1(const uint8_t *message, uint32_t len, uint8_t *hash) {
uint32_t h[5] = {0x67452301, 0xEFCDAB89, 0x98BADCFE, 0x10325476, 0xC3D2E1F0};
uint32_t w[80];
uint32_t a, b, c, d, e, f, k, temp;
for (int i = 0; i < len / 64; i++) {
// 将消息分块
for (int j = 0; j < 16; j++) {
w[j] = (message[i*64 + j*4] << 24) | (message[i*64 + j*4 + 1] << 16) | (message[i*64 + j*4 + 2] << 8) | (message[i*64 + j*4 + 3]);
}
for (int j = 16; j < 80; j++) {
w[j] = (w[j-3] ^ w[j-8] ^ w[j-14] ^ w[j-16]);
w[j] = (w[j] << 1) | (w[j] >> 31);
}
// 初始化变量
a = h[0], b = h[1], c = h[2], d = h[3], e = h[4];
// 消息扩展和算法迭代
for (int j = 0; j < 80; j++) {
if (j < 20) {
f = (b & c) | (~b & d);
k = 0x5A827999;
} else if (j < 40) {
f = b ^ c ^ d;
k = 0x6ED9EBA1;
} else if (j < 60) {
f = (b & c) | (b & d) | (c & d);
k = 0x8F1BBCDC;
} else {
f = b ^ c ^ d;
k = 0xCA62C1D6;
}
temp = (a << 5) | (a >> 27);
temp += f + e + k + w[j];
e = d;
d = c;
c = (b << 30) | (b >> 2);
b = a;
a = temp;
}
// 更新哈希值
h[0] += a;
h[1] += b;
h[2] += c;
h[3] += d;
h[4] += e;
}
// 输出哈希值
for (int i = 0; i < 5; i++) {
hash[i*4] = (h[i] >> 24) & 0xFF;
hash[i*4 + 1] = (h[i] >> 16) & 0xFF;
hash[i*4 + 2] = (h[i] >> 8) & 0xFF;
hash[i*4 + 3] = h[i] & 0xFF;
}
}
int main() {
uint8_t message[] = "Hello, world!";
uint8_t hash[SHA1_BLOCK_SIZE];
sha1(message, strlen(message), hash);
printf("Message: %s\n", message);
printf("Hash: ");
for (int i = 0; i < SHA1_BLOCK_SIZE; i++) {
printf("%02x", hash[i]);
}
printf("\n");
return 0;
}
```
该代码将输入的消息分块、进行消息扩展和算法迭代,最终输出20字节的哈希值。需要注意的是,该代码并不是SHA-1算法的最优实现,仅供参考。
阅读全文
相关推荐
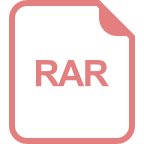
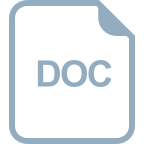

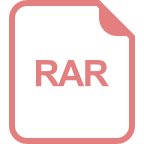
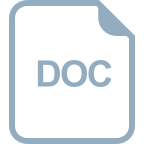
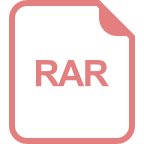
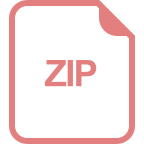
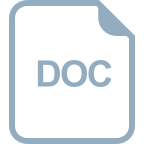
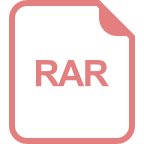
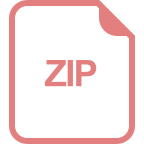
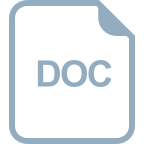
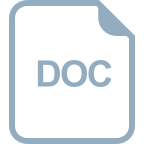

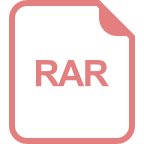
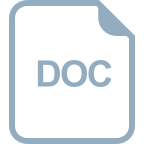
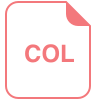