select函数怎么设置串口
时间: 2024-05-13 13:17:50 浏览: 154
在使用select函数进行串口的读写时,需要将串口文件描述符(通常为整数)添加到select函数的监视对象集合中。在调用select函数之前,需要首先打开串口并设置其参数,例如波特率、数据位、停止位等。一般情况下,可以通过以下步骤设置串口:
1. 打开串口:使用open函数打开串口文件,例如 /dev/ttyS0。
2. 设置串口参数:使用tcsetattr函数设置串口的参数,例如波特率、数据位、停止位等。
3. 将串口文件描述符添加到select函数的监视对象集合中。
4. 使用select函数进行读写操作:在监视对象集合中有数据可读或可写时,select函数会返回,此时可以使用read或write函数进行读写操作。
下面是一个简单的示例代码,演示如何使用select函数进行串口读写:
```
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
#include <sys/select.h>
int main()
{
int fd;
struct termios options;
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NONBLOCK);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
// 设置串口参数
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
// 将串口文件描述符添加到监视对象集合中
fd_set readfds, writefds;
FD_ZERO(&readfds);
FD_ZERO(&writefds);
FD_SET(fd, &readfds);
FD_SET(fd, &writefds);
while (1) {
// 使用select函数进行读写操作
select(fd + 1, &readfds, &writefds, NULL, NULL);
if (FD_ISSET(fd, &readfds)) {
char buf[1024];
int len = read(fd, buf, sizeof(buf));
if (len > 0) {
// 处理读取到的数据
printf("Received %d bytes: %.*s\n", len, len, buf);
}
}
if (FD_ISSET(fd, &writefds)) {
char msg[] = "Hello, world!";
int len = write(fd, msg, sizeof(msg));
if (len > 0) {
// 处理已发送的数据
printf("Sent %d bytes: %.*s\n", len, len, msg);
}
}
}
close(fd);
return 0;
}
```
阅读全文
相关推荐
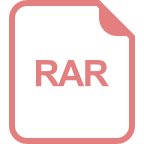
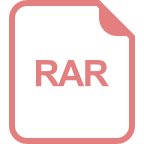
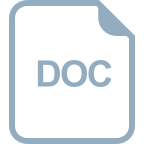
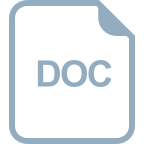
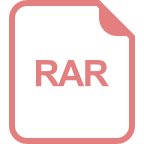
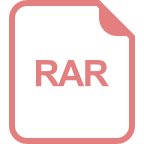
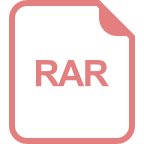
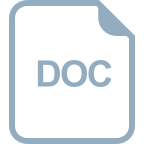
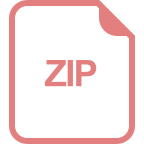
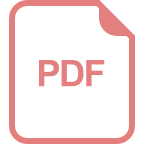
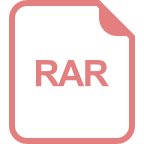
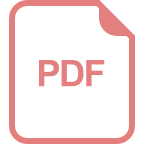
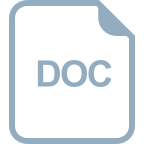



