springboot 生成二维码 并用扫码器扫描完成签到
时间: 2023-07-06 17:41:26 浏览: 163
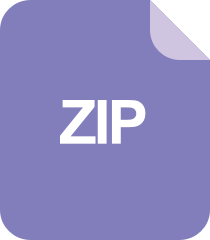
Spring Boot Web 生成并显示二维码
好的,针对您的问题,可以使用以下步骤来实现:
1. 添加依赖:在 pom.xml 中添加以下依赖:
```xml
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.4.1</version>
</dependency>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>javase</artifactId>
<version>3.4.1</version>
</dependency>
```
2. 创建二维码:使用以下代码生成二维码图片并保存到本地:
```java
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
public class QRCodeUtil {
/**
* 生成二维码
*
* @param content 二维码内容
* @param width 二维码宽度
* @param height 二维码高度
* @param format 二维码格式
* @param outputFile 二维码输出文件
* @throws WriterException
* @throws IOException
*/
public static void createQRCode(String content, int width, int height, String format, File outputFile)
throws WriterException, IOException {
HashMap<EncodeHintType, Object> hints = new HashMap<>();
hints.put(EncodeHintType.CHARACTER_SET, "UTF-8");
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.M);
hints.put(EncodeHintType.MARGIN, 2);
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, width, height, hints);
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, bitMatrix.get(x, y) ? 0xFF000000 : 0xFFFFFFFF);
}
}
ImageIO.write(image, format, outputFile);
}
}
```
3. 将二维码图片展示给用户:在 Spring Boot 中可以使用 Thymeleaf 模板引擎来展示二维码图片,具体代码如下:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Sign In</title>
<meta charset="UTF-8"/>
<meta name="viewport" content="width=device-width, initial-scale=1.0"/>
</head>
<body>
<div>
<img th:src="@{'file://' + ${qrCodeFile.getAbsolutePath()}}" th:alt="二维码"/>
</div>
</body>
</html>
```
4. 扫描二维码:用户使用扫码器扫描二维码,获取二维码中的内容,完成签到操作。
以上是实现 Spring Boot 生成二维码并用扫码器扫描完成签到的基本步骤,具体实现还需要根据实际业务场景进行调整。
阅读全文
相关推荐
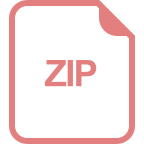
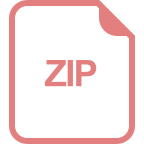
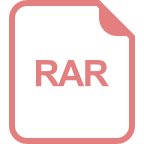
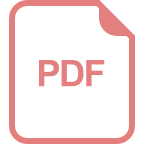
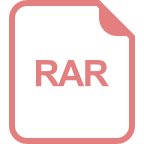
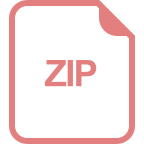
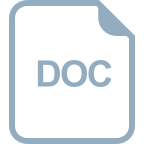

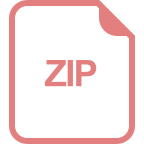
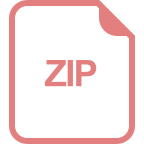
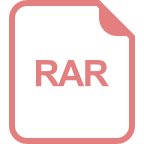
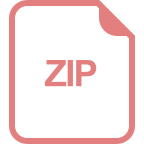
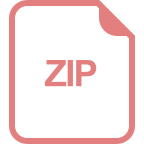
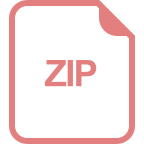
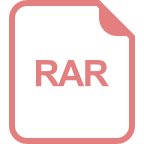
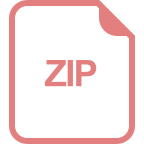
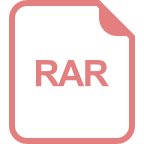