package shiyan; import java.util.Comparator; import java.util.TreeSet; class MyComparator implements Comparator<String> { public int compare(String s1, String s2) { return s1.length() - s2.length(); } } public class ComparatorDemo { public static void main(String[] args) { TreeSet<String> ts = new TreeSet<>(new MyComparator()); ts.add("Jack"); ts.add("Helena"); ts.add("Eve"); System.out.println(ts); } }分析此代码
时间: 2024-04-06 19:29:50 浏览: 69
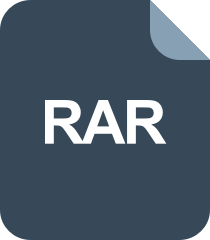
shiyan.rar_Rpt_Hour_shiyan.58.vom
这段代码实现了一个自定义比较器 `MyComparator`,用来比较 `String` 类型的对象。`MyComparator` 类实现了 `Comparator<String>` 接口,重写了 `compare` 方法,比较两个字符串的长度。
在 `main` 方法中,创建了一个 `TreeSet<String>` 对象 `ts`,并传入 `MyComparator` 对象作为参数,这样 `ts` 中的元素会按照字符串长度从小到大排序。然后向 `ts` 中添加了三个字符串 "Jack","Helena","Eve",最后输出 `ts`,结果为 ["Eve", "Jack", "Helena"],符合预期。
阅读全文
相关推荐
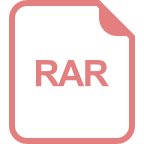
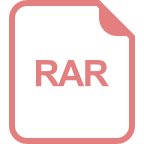















