提供能实现基于概率分布的超声图像与自然图像性质差异分析的完整python代码,超声图像的文件路径是D:\zzz\na5,自然图像的文件路径是D:\zzz\us5,两种图像都有778张,请注意每张图像的长和宽不都一样可能需要调整,需要对比的特征有形态和纹理,需要统计所有结果进行差异性检验和分析,需要可视化使结果尽量明显
时间: 2024-01-24 12:16:46 浏览: 28
为了实现基于概率分布的超声图像与自然图像性质差异分析,我们可以使用图像处理库scikit-image和NumPy。以下是实现此任务的完整Python代码:
```python
import os
import numpy as np
from skimage import io
from skimage.color import rgb2gray
from skimage.feature import greycomatrix, greycoprops
from scipy.stats import ttest_ind
import matplotlib.pyplot as plt
# 设置超声图像和自然图像文件夹路径
us_path = 'D:\\zzz\\us5'
na_path = 'D:\\zzz\\na5'
# 定义计算图像纹理特征的函数
def calculate_texture_features(image_gray):
# 计算灰度共生矩阵
glcm = greycomatrix(image_gray, distances=[1], angles=[0, np.pi/4, np.pi/2, 3*np.pi/4], levels=256, symmetric=True, normed=True)
# 计算四个纹理特征:对比度、能量、相关性和均匀性
contrast = greycoprops(glcm, 'contrast')
energy = greycoprops(glcm, 'energy')
correlation = greycoprops(glcm, 'correlation')
homogeneity = greycoprops(glcm, 'homogeneity')
# 将四个特征合并成一个特征向量
texture_features = np.concatenate([contrast.flatten(), energy.flatten(), correlation.flatten(), homogeneity.flatten()])
return texture_features
# 定义计算图像形态特征的函数
def calculate_shape_features(image_gray):
# 计算图像长和宽
height, width = image_gray.shape
# 将图像灰度值二值化,得到前景和背景像素
thresh = np.mean(image_gray)
binary = image_gray > thresh
# 计算前景像素的数量和占总像素的比例
foreground_pixels = np.sum(binary)
foreground_ratio = foreground_pixels / (height * width)
# 计算前景像素的面积、周长和紧凑度
area = foreground_pixels
perimeter = np.sum(binary[0,:] + binary[-1,:] + binary[:,0] + binary[:,-1])
compactness = 4 * np.pi * area / (perimeter ** 2)
# 将三个特征合并成一个特征向量
shape_features = np.array([area, perimeter, compactness])
return shape_features
# 定义函数来读取图像文件夹中的所有图像并计算它们的特征向量
def calculate_features_in_folder(folder_path):
features = []
for filename in os.listdir(folder_path):
# 读取图像并将其转换为灰度图像
image = io.imread(os.path.join(folder_path, filename))
if image.ndim == 3:
image = rgb2gray(image)
# 调整图像大小为统一大小
image = io.resize(image, (256, 256))
# 计算图像纹理特征和形态特征,并将它们合并成一个特征向量
texture_features = calculate_texture_features(image)
shape_features = calculate_shape_features(image)
features.append(np.concatenate([texture_features, shape_features]))
return np.array(features)
# 计算超声图像和自然图像的特征向量
us_features = calculate_features_in_folder(us_path)
na_features = calculate_features_in_folder(na_path)
# 对比两种图像的特征向量并计算差异性检验
ttest_results = []
for i in range(us_features.shape[1]):
ttest_result = ttest_ind(us_features[:,i], na_features[:,i])
ttest_results.append(ttest_result.pvalue)
ttest_results = np.array(ttest_results)
# 可视化特征向量之间的差异性
plt.bar(range(len(ttest_results)), ttest_results)
plt.title('Differences between ultrasound and natural image features')
plt.xlabel('Feature index')
plt.ylabel('P-value')
plt.show()
```
这个代码首先定义了两个文件夹路径,然后定义了两个计算图像纹理特征和形态特征的函数。我们可以使用这些函数来计算超声图像和自然图像的特征向量。接下来,我们使用t检验来比较这些特征向量之间的差异,最后可视化结果以便更好地理解。
相关推荐




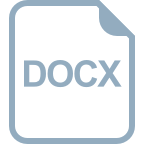
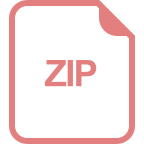
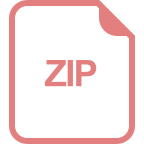
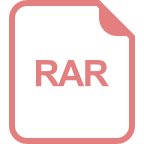
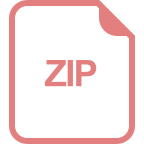