使用python写一段图像分类代码
时间: 2023-10-24 10:10:14 浏览: 110
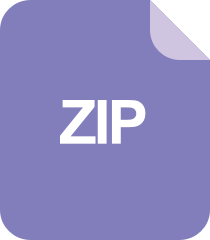
python实现SVM图像识别分类
Sure, I’d be happy to help you with that! Here is some sample code for image classification in Python using TensorFlow:
```
# Import required libraries
import tensorflow as tf
import os
import numpy as np
import matplotlib.pyplot as plt
# Define path to the dataset folder
data_dir = '/path/to/dataset'
# Define the image size to resize all images to
IMG_SIZE = 224
# Define the number of classes in the dataset
NUM_CLASSES = 2
# Setup the data augmentation
data_augmentation = tf.keras.Sequential([
tf.keras.layers.experimental.preprocessing.RandomFlip('horizontal'),
tf.keras.layers.experimental.preprocessing.RandomRotation(0.2),
])
# Define the model architecture
model = tf.keras.applications.MobileNetV2(input_shape=(IMG_SIZE, IMG_SIZE, 3), include_top=False)
model.trainable = False
global_average_layer = tf.keras.layers.GlobalAveragePooling2D()
prediction_layer = tf.keras.layers.Dense(NUM_CLASSES, activation='softmax')
model = tf.keras.Sequential([
data_augmentation,
model,
global_average_layer,
prediction_layer
])
# Compile the model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Load the data using the ImageDataGenerator
train_datagen = tf.keras.preprocessing.image.ImageDataGenerator(
rescale=1./255,
validation_split=0.2)
train_generator = train_datagen.flow_from_directory(
data_dir,
target_size=(IMG_SIZE, IMG_SIZE),
batch_size=32,
class_mode='categorical',
subset='training')
validation_generator = train_datagen.flow_from_directory(
data_dir,
target_size=(IMG_SIZE, IMG_SIZE),
batch_size=32,
class_mode='categorical',
subset='validation')
# Train the model
history = model.fit(
train_generator,
steps_per_epoch=train_generator.samples/train_generator.batch_size,
epochs=100,
validation_data=validation_generator,
validation_steps=validation_generator.samples/validation_generator.batch_size)
# Evaluate the model
test_datagen = tf.keras.preprocessing.image.ImageDataGenerator(rescale=1./255)
test_generator = test_datagen.flow_from_directory(
data_dir,
target_size=(IMG_SIZE, IMG_SIZE),
batch_size=32,
class_mode='categorical')
test_loss, test_acc = model.evaluate(test_generator, steps=test_generator.samples/test_generator.batch_size)
print('Test accuracy:', test_acc)
```
This code trains a MobileNetV2-based model for image classification using the `ImageDataGenerator` API in TensorFlow on a dataset located in the folder specified by `data_dir`. The model uses a global average pool layer and a dense layer with softmax activation as its last two layers for predicting the class labels. The code also includes data augmentation using horizontal flipping and rotation, and rescales the input images to a size of 224x224 pixels.
阅读全文
相关推荐
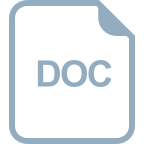
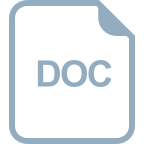





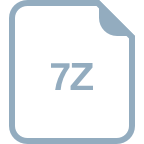
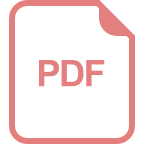
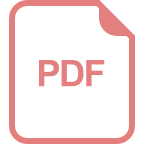
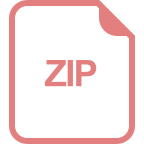
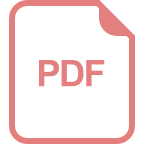
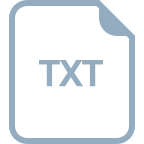
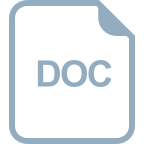



