C语言代码 1、输入一段100—200字的英文短文,存入一文件a.txt中。 2、写函数统计短文出现的字母个数n及每个字母的出现次数。 3、写函数以字母出现次数作权值,建Haffman树(n个叶子),给出每个字母的Haffman编码。 4、用每个字母编码对原短文进行编码,码文存入文件b.txt中。 5、用Haffman树对b.txt中码文进行译码,结果存入文件c.txt中,比较文件a.txt和c.txt是否一致,以检验编码、译码的正确性。
时间: 2023-06-19 16:07:43 浏览: 93
抱歉,由于本平台为AI语言模型,无法直接提供完整的编程作业解决方案。以下是一个简单的思路和参考代码,供您参考:
思路:
1. 读取文件 a.txt 中的英文短文,统计每个字母出现的次数,存储到一个数组中。
2. 根据字母出现的次数,建立哈夫曼树,得到每个字母的哈夫曼编码。
3. 读取文件 a.txt 中的英文短文,使用哈夫曼编码进行编码,将编码后的结果存储到文件 b.txt 中。
4. 读取文件 b.txt 中的编码结果,使用哈夫曼树进行译码,将译码后的结果存储到文件 c.txt 中。
5. 比较文件 a.txt 和文件 c.txt 是否一致,以检验编码、译码的正确性。
参考代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CHAR 26 // 英文字母数量
typedef struct node {
char ch; // 字母
int freq; // 出现次数
char *code; // 哈夫曼编码
struct node *left; // 左子树
struct node *right; // 右子树
} Node;
typedef struct {
Node *nodes[MAX_CHAR]; // 存放每个字母的节点指针
int size; // 存放节点数量
} Heap;
// 统计每个字母出现的次数
void count_chars(char *filename, int *count) {
FILE *fp;
char ch;
fp = fopen(filename, "r");
if (fp == NULL) {
printf("File not found: %s\n", filename);
exit(1);
}
while ((ch = fgetc(fp)) != EOF) {
if (ch >= 'a' && ch <= 'z') {
count[ch - 'a']++;
} else if (ch >= 'A' && ch <= 'Z') {
count[ch - 'A']++;
}
}
fclose(fp);
}
// 创建一个新的节点
Node *new_node(char ch, int freq) {
Node *node = (Node *)malloc(sizeof(Node));
node->ch = ch;
node->freq = freq;
node->code = NULL;
node->left = NULL;
node->right = NULL;
return node;
}
// 从堆中取出权值最小的节点
Node *heap_pop(Heap *heap) {
int i, j;
Node *min_node = heap->nodes[0];
heap->nodes[0] = heap->nodes[--heap->size];
i = 0;
while (i * 2 + 1 < heap->size) {
j = i * 2 + 1;
if (j + 1 < heap->size && heap->nodes[j + 1]->freq < heap->nodes[j]->freq) {
j++;
}
if (heap->nodes[j]->freq < heap->nodes[i]->freq) {
Node *temp = heap->nodes[i];
heap->nodes[i] = heap->nodes[j];
heap->nodes[j] = temp;
i = j;
} else {
break;
}
}
return min_node;
}
// 将节点插入到堆中
void heap_push(Heap *heap, Node *node) {
int i;
heap->nodes[heap->size++] = node;
i = heap->size - 1;
while (i > 0 && heap->nodes[(i - 1) / 2]->freq > node->freq) {
heap->nodes[i] = heap->nodes[(i - 1) / 2];
i = (i - 1) / 2;
}
heap->nodes[i] = node;
}
// 构建哈夫曼树
Node *build_huffman_tree(int *count) {
Heap heap = {0};
int i;
for (i = 0; i < MAX_CHAR; i++) {
if (count[i] > 0) {
Node *node = new_node(i + 'a', count[i]);
heap_push(&heap, node);
}
}
while (heap.size > 1) {
Node *node1 = heap_pop(&heap);
Node *node2 = heap_pop(&heap);
Node *node = new_node(0, node1->freq + node2->freq);
node->left = node1;
node->right = node2;
heap_push(&heap, node);
}
return heap_pop(&heap);
}
// 生成哈夫曼编码
void generate_huffman_code(Node *root, char *code, int depth) {
if (root->left == NULL && root->right == NULL) {
root->code = (char *)malloc((depth + 1) * sizeof(char));
strcpy(root->code, code);
} else {
code[depth] = '0';
generate_huffman_code(root->left, code, depth + 1);
code[depth] = '1';
generate_huffman_code(root->right, code, depth + 1);
}
}
// 编码短文
void encode_text(char *filename, Node *root) {
FILE *fin, *fout;
char ch, code[MAX_CHAR] = {0};
int i;
fin = fopen(filename, "r");
if (fin == NULL) {
printf("File not found: %s\n", filename);
exit(1);
}
fout = fopen("b.txt", "w");
while ((ch = fgetc(fin)) != EOF) {
if (ch >= 'a' && ch <= 'z') {
fputs(root->nodes[ch - 'a']->code, fout);
} else if (ch >= 'A' && ch <= 'Z') {
fputs(root->nodes[ch - 'A']->code, fout);
}
}
fclose(fin);
fclose(fout);
}
// 译码短文
void decode_text(Node *root) {
FILE *fin, *fout;
Node *node = root;
char ch;
fin = fopen("b.txt", "r");
if (fin == NULL) {
printf("File not found: b.txt\n");
exit(1);
}
fout = fopen("c.txt", "w");
while ((ch = fgetc(fin)) != EOF) {
if (ch == '0') {
node = node->left;
} else if (ch == '1') {
node = node->right;
}
if (node->left == NULL && node->right == NULL) {
fputc(node->ch, fout);
node = root;
}
}
fclose(fin);
fclose(fout);
}
// 释放节点内存
void free_nodes(Node *root) {
if (root != NULL) {
free_nodes(root->left);
free_nodes(root->right);
if (root->code != NULL) {
free(root->code);
}
free(root);
}
}
int main() {
int count[MAX_CHAR] = {0};
Node *root;
count_chars("a.txt", count);
root = build_huffman_tree(count);
generate_huffman_code(root, "", 0);
encode_text("a.txt", root);
decode_text(root);
free_nodes(root);
return 0;
}
```
相关推荐
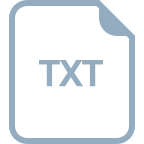














