springboot远程控制linux
时间: 2023-11-11 17:00:00 浏览: 72
可以使用SSH协议来远程控制Linux,Spring Boot中可以使用JSch库来实现SSH连接和命令执行。具体步骤如下:
1. 引入JSch库的依赖:
```xml
<dependency>
<groupId>com.jcraft</groupId>
<artifactId>jsch</artifactId>
<version>0.1.55</version>
</dependency>
```
2. 编写SSH连接和命令执行的代码:
```java
public class SSHUtil {
private static final Logger logger = LoggerFactory.getLogger(SSHUtil.class);
private String host;
private int port;
private String username;
private String password;
public SSHUtil(String host, int port, String username, String password) {
this.host = host;
this.port = port;
this.username = username;
this.password = password;
}
public void executeCommand(String command) throws Exception {
JSch jsch = new JSch();
Session session = jsch.getSession(username, host, port);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
ChannelExec channelExec = (ChannelExec) session.openChannel("exec");
channelExec.setCommand(command);
channelExec.setInputStream(null);
channelExec.setErrStream(System.err);
InputStream in = channelExec.getInputStream();
channelExec.connect();
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
String line;
while ((line = reader.readLine()) != null) {
logger.info(line);
}
channelExec.disconnect();
session.disconnect();
}
}
```
3. 在Spring Boot中使用SSHUtil类:
```java
@RestController
public class SSHController {
@GetMapping("/executeCommand")
public String executeCommand() {
try {
SSHUtil sshUtil = new SSHUtil("192.168.1.100", 22, "root", "password");
sshUtil.executeCommand("ls -l");
return "Command executed successfully!";
} catch (Exception e) {
return "Failed to execute command: " + e.getMessage();
}
}
}
```
阅读全文
相关推荐







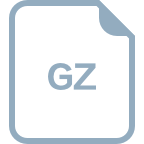








