3、代码填空:设计一个能存储任意类型数据的顺序堆栈,即设计一个堆栈模板,然后用某种类型的数据进行验证,并进行进栈和出栈操作。 背景知识:堆栈是一种存储结构,它的特点是先进后出结构,堆栈中有一个栈顶指针,始终指向栈顶上的元素。程序中,建立的一个顺序栈,采用数组。 #include <iostream> #define MAXSIZE 50 using namespace std; template <class T> { private: T s[MAXSIZE]; int top; public: stack() {top=-1;} void push(T newvalue); //进栈 T pop( ); //出栈 }; void stack<T>::push( T newvalue) { if ( ) { top=top+1; s[top]=newvlaue; } else cout<<”栈满,无法进栈!”<<endl; } template <class T> T ::pop() { if (top>-1) { cout<<s[top]<<endl; top=top-1; } else cout<<”堆栈已空!无法出栈!”<<endl; } void main( ) { stack< > args; args.push(10); args.push(20); args.pop( ); args.pop( ); args.pop( ); } 请回答问题: (1)为何要定义栈? 栈的代码实现是依赖数组,是如何实现数组“先进后出”特性的?
时间: 2024-02-04 10:04:26 浏览: 32
代码填空:
```cpp
#include <iostream>
#define MAXSIZE 50
using namespace std;
template <class T>
class stack {
private:
T s[MAXSIZE];
int top;
public:
stack() {top=-1;}
void push(T newvalue); //进栈
T pop( ); //出栈
};
template <class T>
void stack<T>::push( T newvalue) {
if (top < MAXSIZE - 1) {
top++;
s[top] = newvalue;
} else {
cout << "栈满,无法进栈!" << endl;
}
}
template <class T>
T stack<T>::pop() {
if (top > -1) {
T temp = s[top];
top--;
return temp;
} else {
cout << "堆栈已空!无法出栈!" << endl;
return T();
}
}
int main() {
stack<int> args;
args.push(10);
args.push(20);
cout << args.pop() << endl;
cout << args.pop() << endl;
cout << args.pop() << endl;
return 0;
}
```
(1) 定义栈的主要目的是用于后续进行数据的存储和访问,具体来说,栈在计算机程序中被广泛应用,例如函数调用、表达式求值、内存分配等。
栈的代码实现是依赖数组,实现数组“先进后出”特性的原理是栈的元素只能在栈顶进行插入和删除操作,其次,栈的元素必须按照“后进先出”的原则进行访问,即先进入栈的元素只有在后进入栈的元素出栈后才能访问。因此,每次进栈时,将元素插入到栈顶,每次出栈时,从栈顶取出元素。这样就能实现数组“先进后出”的特性。
相关推荐
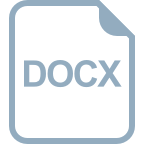
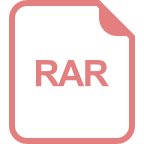
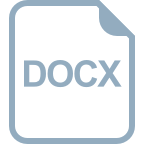
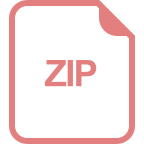
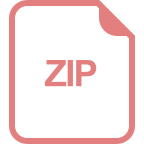
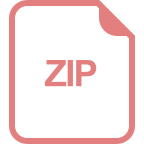
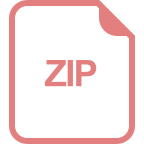
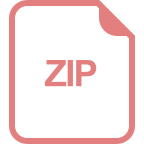