stack在c语言中怎么用
时间: 2023-09-08 13:16:25 浏览: 54
在C语言中,stack通常指的是栈(stack)数据结构。C语言中可以使用数组或者链表来实现栈。
使用数组实现栈的基本操作:
1.定义一个数组和一个变量top表示栈顶位置
```c
#define MAX_SIZE 100
int stack[MAX_SIZE];
int top = -1;
```
2.入栈操作:将元素插入到栈顶位置,并将top指针加1
```c
void push(int x) {
if (top == MAX_SIZE - 1) {
printf("error: stack overflow\n");
return;
}
stack[++top] = x;
}
```
3.出栈操作:将栈顶元素弹出,并将top指针减1
```c
int pop() {
if (top == -1) {
printf("error: stack underflow\n");
return -1;
}
return stack[top--];
}
```
4.获取栈顶元素:返回栈顶元素,但不弹出
```c
int peek() {
if (top == -1) {
printf("error: stack underflow\n");
return -1;
}
return stack[top];
}
```
使用链表实现栈的基本操作:
1.定义一个链表结构体和一个指向栈顶的指针top
```c
typedef struct node {
int data;
struct node *next;
} Node;
Node *top = NULL;
```
2.入栈操作:创建一个新的节点,将其插入到链表头部,并将top指向该节点
```c
void push(int x) {
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->data = x;
new_node->next = top;
top = new_node;
}
```
3.出栈操作:将链表头部节点删除,并将top指向下一个节点
```c
int pop() {
if (top == NULL) {
printf("error: stack underflow\n");
return -1;
}
Node *temp = top;
int data = temp->data;
top = top->next;
free(temp);
return data;
}
```
4.获取栈顶元素:返回栈顶元素,但不弹出
```c
int peek() {
if (top == NULL) {
printf("error: stack underflow\n");
return -1;
}
return top->data;
}
```
相关推荐
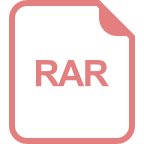
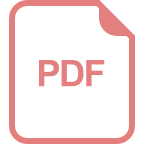
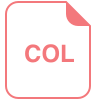
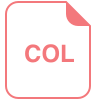
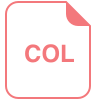
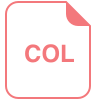
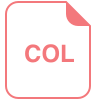









