自定义一个日期类,有年月日三个私有成员变量,再写set和get方法来设置或访问它们的值,另外写一个display方法打印出他们的值。然后在一个main方法中测试一下Java
时间: 2024-10-01 09:07:07 浏览: 37
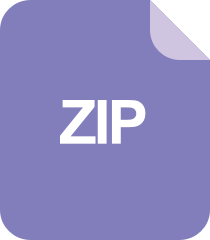
Dash-Calendar:一个日历可以使用多个日期选择器功能
在Java中,你可以自定义一个日期类(通常我们会创建一个`Date`或`DateTime`类型的类,因为Java内置了`java.time`包下的`LocalDate`、`LocalDateTime`等类),如下所示:
```java
public class CustomDate {
private int year;
private int month;
private int day;
// 构造函数
public CustomDate(int year, int month, int day) {
this.year = year;
this.month = month;
this.day = day;
}
// Setters (设置方法)
public void setYear(int year) {
this.year = year;
}
public void setMonth(int month) {
if(month >= 1 && month <= 12) { // 验证月份范围
this.month = month;
} else {
throw new IllegalArgumentException("Invalid month");
}
}
public void setDay(int day) {
if(day >= 1 && day <= getDaysInMonth()) { // 获取月份天数并验证范围
this.day = day;
} else {
throw new IllegalArgumentException("Invalid day");
}
}
// Getters (获取方法)
public int getYear() {
return year;
}
public int getMonth() {
return month;
}
public int getDay() {
return day;
}
// display method (打印方法)
public String display() {
return "Year: " + year + ", Month: " + month + ", Day: " + day;
}
// Helper method to calculate days in a month
private int getDaysInMonth() {
int[] daysInMonth = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if(isLeapYear(year)) { // 判断是否为闰年
return daysInMonth[month];
} else {
return daysInMonth[month - 1]; // February has 28 days in non-leap years
}
}
// Leap year check
private boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
}
// 测试main方法
public static void main(String[] args) {
CustomDate customDate = new CustomDate(2023, 2, 25);
customDate.setYear(2024); // 设置新的年份
customDate.setMonth(7); // 设置新的月份
customDate.setDay(1); // 设置新的日期
System.out.println(customDate.display()); // 输出当前日期
}
```
在这个例子中,我们创建了一个名为`CustomDate`的类,并实现了年、月、日的设置和获取方法以及`display()`方法用于显示日期信息。`getDaysInMonth()`是一个辅助方法来计算给定月份的天数,而`isLeapYear()`则判断是否为闰年。在`main`方法中,我们实例化这个类并进行了简单的设置和测试。
阅读全文
相关推荐
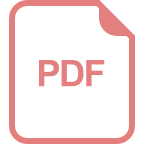
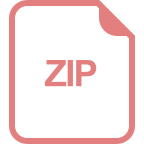














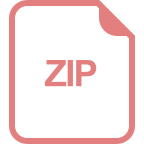
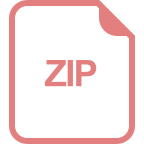